Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial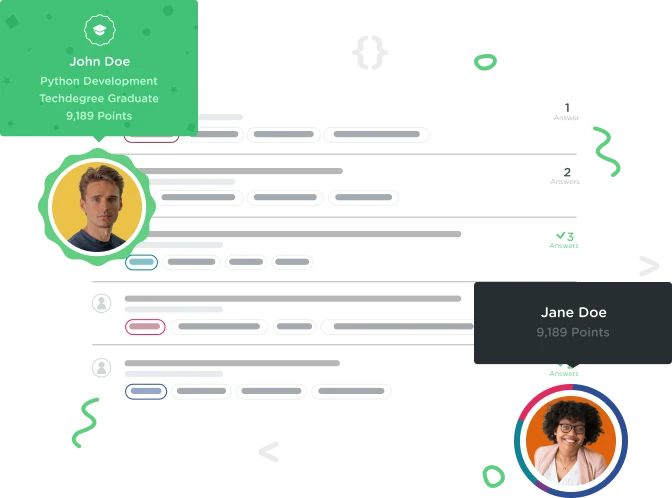
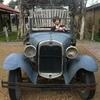
Brendan Moran
14,052 PointsIs it ok to use a method to get a state *IF* I never, ever want to set that state?
Just messing around with different possible solutions, I've got some utility methods in my book class -- isOverdue() and daysOverdue() -- because I thought it made more sense to have this information here than only in the chargeFines() method in the library class. These methods are technically giving back state and not really "doing" any kind of behavior, so they should technically be properties, I guess. But I can't think of any situation where I would want to allow any user to manually set these as properties using a setter. I only see that further complicating the code, creating the need for more conditionals, and creating room for more errors. Anyway, here's the code. More questions at the bottom.
class Book {
constructor(title, author, isbn) {
this.title = title;
this.author = author;
this.isbn = isbn;
this.patron = null;
this.dueDate = null;
this._checkedOut = false;
}
static newDueDate() {
const date = new Date();
date.setDate(date.getDate() + 14);
return date;
}
set checkedOut(bool) {
this._checkedOut = bool;
if (bool) {
this.dueDate = Book.newDueDate();
} else {
this.dueDate = null;
}
}
get checkedOut() {
return this._checkedOut;
}
isOverdue() {
const now = new Date();
if (this.dueDate && this.dueDate < now) {
return true;
} else {
return false;
}
}
daysOverdue() {
const now = new Date();
if (this.dueDate && this.dueDate < now) {
const daysOverdue = Math.floor((now - this.dueDate) / 86400000);
return daysOverdue;
} else {
return 0;
}
}
}
These methods are then used in the library class, like this:
chargeFines() {
this.patrons.forEach((patron) => {
let book = patron.currentBook;
if (book.isOverdue()) {
patron.balance +=
Math.ceil(this.dailyFine * book.daysOverdue() * 100) / 100;
book.dueDate = new Date();
}
});
So, is this ok? Or are these methods in the book class a Very Bad Thingβ’?
Should I turn them into static methods? Or should I really do setters/getters for each one? I really can't see a case where I would want a user to manually set a book.isOverdue or book.daysOverdue property. I only see the need to calculate and retrieve them dynamically.
1 Answer
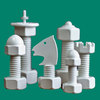
Steven Parker
231,275 PointsAnything that references "this" is probably better implemented as an instance method rather than a static one.
And you can certainly have read-only properties. If you convert them you only need to implement the "get" method.
Brendan Moran
14,052 PointsBrendan Moran
14,052 PointsAh-hah. So I can just do a getter and no setter (wasn't sure if that was bad practice, or what). Good to know!
Do you think making them getters is preferable to having them as methods, or is just whatever floats yer boat?
Steven Parker
231,275 PointsSteven Parker
231,275 PointsIt's purely choice,but if you go with properties you can use them without parentheses.