Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial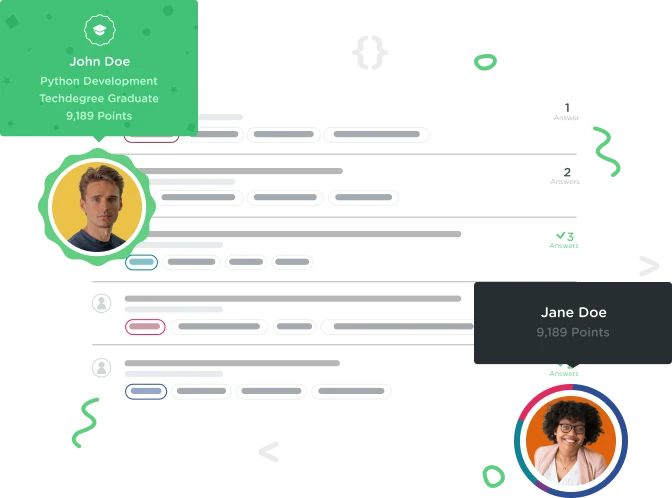

Tyler Brandt
4,729 PointsIs it OK to use correct++ instead of correct += 1?
I used the variable "score" instead of "correct," but instead of using "score += 1," I used "score++" and it worked.
What are the pros and cons of incrementing the variable score like I did.
2 Answers
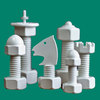
Steven Parker
229,744 PointsIt depends on how you use them.
When you use them as a complete statement in themselves, they do the same thing. But if you were to use them in a formula, assignment, or function call, they would be different.
When placed after the variable name, the ++
operator is known as the "post-increment operator". That means it increments the value after determining what to return. For example, let's say you have a function named "print" that will show a value on the screen. Here's what would happen if you used it on each of these:
var score = 4;
print(score += 1); // this would print "5"
// score is 5 here
var score = 4;
print(score++); // this would print "4"
// score is 5 here also

aiden1408
3,338 PointsWell, when using "variable++", you increment the value by one. But if you use the "variable += [someValue]", you increment the value by any value you'd like. Maybe now you want to increment the variable by only one, but what if later on you decide, that you need to increment with a higher value? Then you have to search for your "variable++" line, and fix it, while if you have the "variable += [otherVariable]", you just change the "otherVariable" and you are done.

Tyler Brandt
4,729 PointsI see! That makes perfect sense. Thank you for taking the time to respond to my question.

Simon Coates
28,694 Pointsthis is true. Soft coding is good practice and is more flexible. However variable++ is typically something you'd see in a loop. In that instance, you may only ever be going forward one, so there no ambiguity about where the implicit 1 comes from. I looked at while loop code examples for c#, Java, and javascript at microsoft, oracle and mozilla respectively. In all instances, the increment syntax was used. (Additionally Steven Parker is right - inevitably - about the pre and postfix distinction. However, it doesn't really matter if the increment is the only thing in a statement.)
Tyler Brandt
4,729 PointsTyler Brandt
4,729 PointsInteresting! Very helpful answer Steven. Yeah, so I tested this myself by doing the following:
In this example, the value logged in the console was 4, but when I typed "score" in the console to see its value, the value of score was 5.