Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial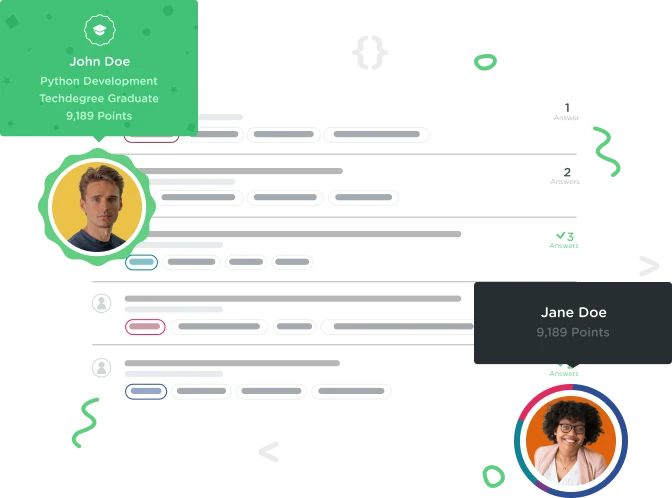

samrat Devkota
Courses Plus Student 4,588 Pointsis it okay to do this? this code also gives the same result as the code taught in the video. What's the difference?
def linear_search(list,target):
for item in list:
if item == target:
return list.index(item)
return None
def verify(index):
if index is not None:
print("Target found at index: ",index)
else:
print("Target not found in the list")
numbers = [1,2,3,4,5,6,7,8,9,10]
result = linear_search(numbers, 6)
verify(result)
1 Answer
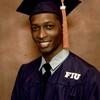
Dane Parchment
Treehouse Moderator 11,077 PointsYes you are fine, at the end of the day a linear search is simply going through each element of the list until you find the first match.
How you traverse through the list is up to you, so long as you can:
- Traverse through each element in the list
- Exit the loop upon finding the first match.
You've done both here, so you're good. you understand the algorithm and can apply it.