Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial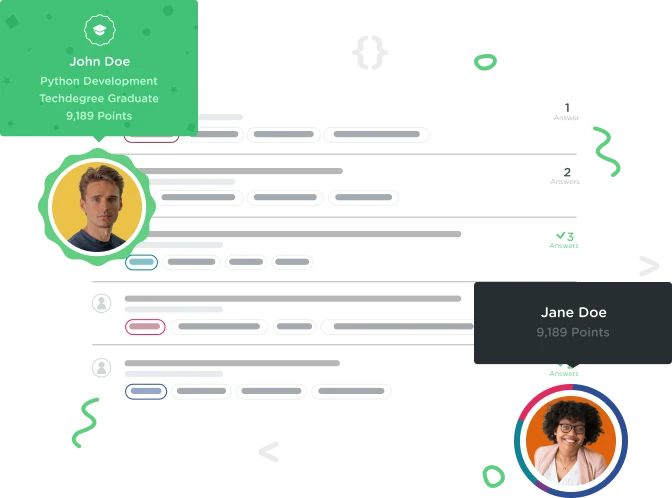
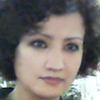
Durdona Abdusamikovna
1,553 PointsIs it possible to compare passed arguments within a function ?
Here is what crossed my mind based on challenge question ...
function randomNumber(top, bottom) {
var randomMath = Math.floor(Math.random() * (top - bottom + 1)) + bottom;
if (parseInt(randomMath) < parseInt(bottom)){
alert("you guessed larger number")
}else {
alert("you guessed smaller number ")
}
}
console.log(randomNumber(100, 20));
Now I want to compare them and write in the document what number was chosen however, my number is always small number and I am missing return value. How can I fix my code if it is ever possible ? Thank you beforehand
4 Answers
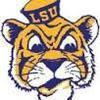
Cena Mayo
55,236 Pointsfunction randomNumber(top, bottom) {
var randomMath = Math.floor(Math.random() * (top - bottom + 1)) + bottom;
if (parseInt(randomMath) > parseInt(bottom)){
alert("you guessed larger number");
}else {
alert("you guessed smaller number ");
}
return randomMath; // or console.log(randomMath);
};
randomNumber(100, 20);
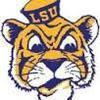
Cena Mayo
55,236 PointsI believe you just need to change your first test to
function randomNumber(top, bottom) {
var randomMath = Math.floor(Math.random() * (top - bottom + 1)) + bottom;
if (parseInt(randomMath) > parseInt(bottom)){
alert("you guessed larger number");
}else {
alert("you guessed smaller number ");
}
};
randomNumber(100, 20);
Please note that, after some (very) limited testing, it still appears that if you choose the same number for both top and bottom, the result will always be 'you guessed smaller number.'
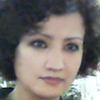
Durdona Abdusamikovna
1,553 PointsI don't see a difference ... in addition in your case you don't have return value as well... I thought that in function we always have to return smth.
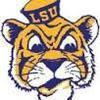
Cena Mayo
55,236 PointsI changed
if (parseInt(randomMath) < parseInt(bottom)){
to
if (parseInt(randomMath) > parseInt(bottom)){
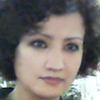
Durdona Abdusamikovna
1,553 Pointshow about return value ... unlike Java in javaScript functions have to have a return value... can we do smth. here again ?
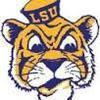
Cena Mayo
55,236 PointsNot sure why the console.log(randomNumber) in your original code was missing from my first post. Anyway, glad to help (and hope I did :)).
Durdona Abdusamikovna
1,553 PointsDurdona Abdusamikovna
1,553 PointsThank you :)