Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial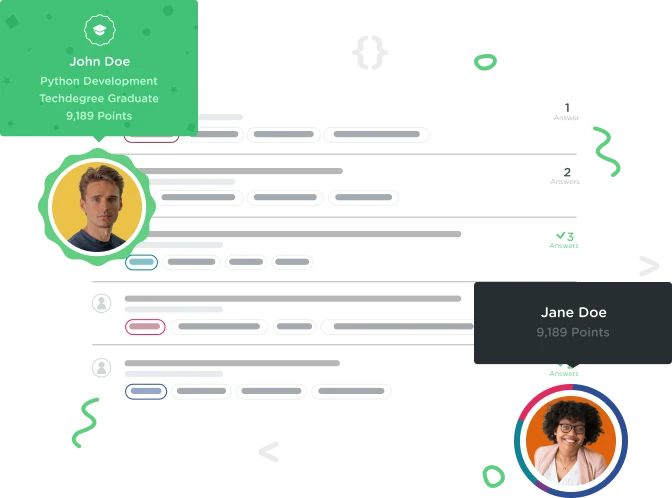
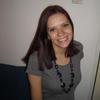
Ivana Lescesen
19,442 PointsIs it possible to do this task by using a while loop and indexOf() method?
Amazing people at treehouse
Is it possible to do this task by using a while
loop and indexOf()
method?
And if not how can we check if the
search = prompt("Search for a student. Type a name of a student or 'quit' to exit");
is equal to
students.name
Thank you so much :)
2 Answers
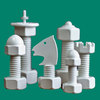
Steven Parker
230,274 PointsThere's two issues with using indexOf in this situation.
- You would have to provide indexOf with the entire student object to match on, not just the name.
- indexOf only finds the first match, but the sample method finds all that have the same name.

Nathan Crum
15,183 PointsInstead of indexOf() you can use the .filter() method on your array that calls a function you create. It then passes each item/object from your array to your function where you should evaluate each item to true or false. For instance:
var array = [
{ name: 'John', points: 22 },
{ name: 'Rebecca', points: 33 },
{ name: 'Thomas', points: 32 },
{ name: 'Elise', points: 10}
];
var bestPlayers = [];
/*
* .filter() passes each object from array to the function
* isScoreHighEnough. My function takes the object and
* tests it against a condition. Any objects that test True
* are returned and any that test false are ignored. The
* array bestPlayers should now contain the two objects
* with Rebecca and Thomas because their points were
* greater than 30.
*
* Reference https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/Array/filter
*/
var bestPlayers = array.filter(isScoreHighEnough);
function isScoreHighEnough(player) {
if(player.points > 30) {
return true;
} else {
return false;
}
}
Ivana Lescesen
19,442 PointsIvana Lescesen
19,442 PointsSteven Parker Thank you so much, what sample method? Would you advise against using index of in this particular task?
And if not how can we check if the
search = prompt("Search for a student. Type a name of a student or 'quit' to exit");
is equal to students.name by using the while loop Thank you so much
Steven Parker
230,274 PointsSteven Parker
230,274 PointsI was referring to the sample method presented in the solution video.
It compares the search term with each student's name in a loop.