Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial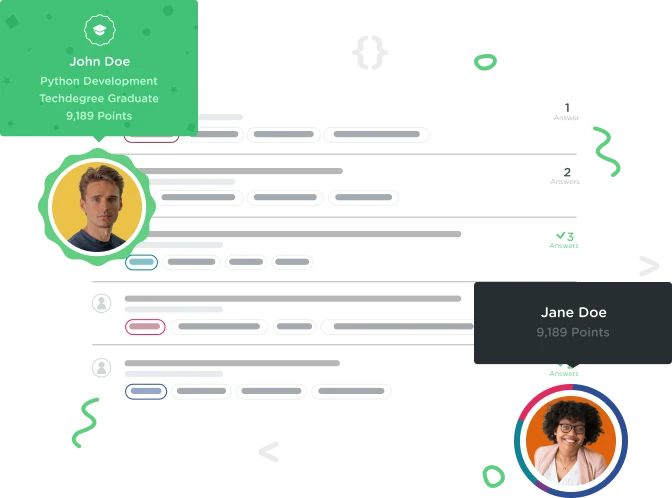
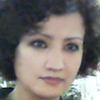
Durdona Abdusamikovna
1,553 PointsIs it possible to ignore space and comma when user answers to a question ?
I could make user's input case insensitive using .toLowerCase() function
answers = quiz[k][1].toLowerCase();
however, can't make comma or space to be ignored. Let's say one of my answer has 'all' and if user put space after it without realizing the answer is wrong. How about another answer when I have "yes it can" and user types "yes, it can" this answer is wrong as well because of comma. How can I resolve this issue ? Thank you beforehand
var quiz = [
["Do you like programming :) ", "YES"],
["Which one is your favourite ? ", "all"],
["It can't be all, can be ?", "yes it can"]
];
2 Answers
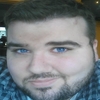
Marcus Parsons
15,719 PointsDurdona! hi! :)
Ali, good call on using regular expressions! I came up with a solution for Durdona based on the use of regular expressions.
So what this code does is replace any occurrence of a character that isn't a lowercase or uppercase alphabet character in both the answer received from the quiz array and the answer received from the user. Both are also transferred into a lower case format. This way, regardless of capitalization or punctuation, the answers can be matched and identified i.e. "yes, it can" in the quiz array and "Yes, it can!" in the response from the user will be identical.
Durdona, regular expressions are among the most difficult concepts to learn but once you do, they are very powerful.
for(var k = 0; k < quiz.length; k += 1){
questions = quiz[k][0];
answers = quiz[k][1].replace(/[^a-zA-Z]/g, '').toLowerCase();
responseInput = prompt(questions).replace(/[^a-zA-Z]/g, '').toLowerCase();
if (responseInput === answers){
guessCounter += 1;
}
}
A good reference point to regular expressions is https://developer.mozilla.org/en-US/docs/Web/JavaScript/Guide/Regular_Expressions and lots of experimentation!
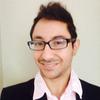
Ali Amirazizi
16,087 PointsTry using escape characters. Set the variable that holds the users input to the escape characters. I haven't verified if this works but I'm interested in the answer.
var str = "B I O S T A L L"
str = str.replace(/ /g,'');
// Outputs 'B I O S T A L L'
var str = "B I O S T A L L"
str = str.replace(/\s/g,'');
// Outputs 'BIOSTALL'
var str = "B I O S T A L L"
str = str.replace(/\s/g,'-');
// Outputs 'B-I-O-S-T-A-L-L'
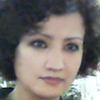
Durdona Abdusamikovna
1,553 PointsThank you Ali for your response. First, I wanted to use escape character which ignores space however, my code already has a different function appended to it maybe my code's approach is not suitable here . Here is the code
for(var k = 0; k < quiz.length; k += 1){
questions = quiz[k][0];
answers = quiz[k][1].toLowerCase();
responseInput = prompt(questions);
if (responseInput === answers){
guessCounter += 1;
}
}
I am targeting only answers's element of the array ....
Durdona Abdusamikovna
1,553 PointsDurdona Abdusamikovna
1,553 PointsI remember learning the same thing ASCII table when I was learning C programming language. As I see most programming languages have the same concept what I saw in C and Java can be similar to JavaScript. It is really helpful to learn a new language faster :) I guess it is just AWESOME and I enjoy learning it :)
Marcus sending you tons of thanks I hope you are strong enough to hold them :) lol
Marcus Parsons
15,719 PointsMarcus Parsons
15,719 PointsI absolutely love learning new languages, as well! I'm about to start on Python here soon on Codeacademy.com then I'm going to switch over to Treehouse.
Well, I can bench right around 250 pounds, so mmmmmm, possibly! haha :D
Durdona Abdusamikovna
1,553 PointsDurdona Abdusamikovna
1,553 PointsCool :)
Marcus Parsons
15,719 PointsMarcus Parsons
15,719 PointsBtw, check out http://www.codeacademy.com if you never have before. It is highly interactive and may help you with JavaScript/jQuery or a multitude of other languages you wish to learn. It is totally free and totally awesome.