Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial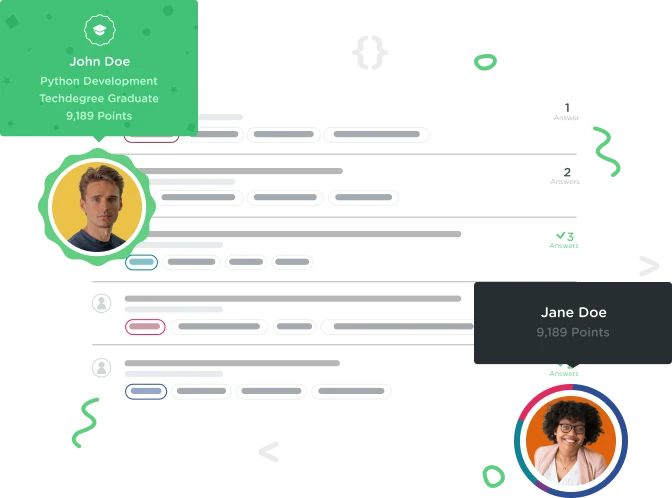
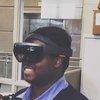
Guled Ahmed
12,806 PointsIs it possible to organize posts by date using Jinja?
I recently tried to implement a system in which posts are grouped by date like so:
Today
post 1
post 2
Yesterday
post 3
post 4
June 2
post 5
nth post
My initial attempts included creating a new Date model with a date property with the default value of datetime.datetime.today() and tried to pass that to my webpage along with the stream and try to make the dates as an outer loop and the stream as an inner loop and tried to evaluate posts under a specific date in this fashion. This method did not work at all. I was wondering if anyone know how to either organize this using database tables or jinja.
Regards, Guled
1 Answer

Dan Johnson
40,532 PointsFor Jinja2 you have sorting options in the form of built-in filters:
You could select whatever date attribute you want to sort on.
For a general solution I threw this together. It's far from optimal but maybe it could give you some ideas for a better one:
Basic Post Model
class Post(Model):
created = DateTimeField(default=datetime.datetime.now)
content = TextField()
@classmethod
def select_posts_from(model, days_ago):
today = datetime.datetime.now()
upper_limit = today - datetime.timedelta(days=days_ago-1)
lower_limit = today - datetime.timedelta(days=days_ago)
clear = {attr: 0 for attr in ["hour","minute","second","microsecond"]}
upper_limit = upper_limit.replace(**clear)
lower_limit = lower_limit.replace(**clear)
upper_query = model.created < upper_limit
lower_query = model.created >= lower_limit
return model.select().where(upper_query & lower_query).order_by(Post.created.desc())
class Meta:
database = DATABASE
Index Route
from collections import OrderedDict
# ...
@app.route("/")
def index():
dates = OrderedDict()
dates["Today"] = Post.select_posts_from(days_ago=0)
dates["Yesterday"] = Post.select_posts_from(days_ago=1)
dates["Two Days Ago"] = Post.select_posts_from(days_ago=2)
return render_template("index.html", dates=dates)
Index Template
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title>Post Sort Example</title>
</head>
<body>
{% for date in dates %}
<h2>{{ date }}</h2>
<ul>
{% for post in dates[date] %}
<li>
<p>{{ post.content }}</p>
<i>Created On: {{ post.created }}</i>
</li>
{% endfor %}
</ul>
{% endfor %}
</body>
</html>
Guled Ahmed
12,806 PointsGuled Ahmed
12,806 PointsThank you for your help Dan. I appreciate it.
Guled Ahmed
12,806 PointsGuled Ahmed
12,806 PointsJinja's groupby feature is the most optimal solution.
Dan Johnson
40,532 PointsDan Johnson
40,532 PointsSwapped to timedeltas for adjustment which should fix any issues with longer ranges of time. Feel free to yell at me if you see anything else broken.