Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial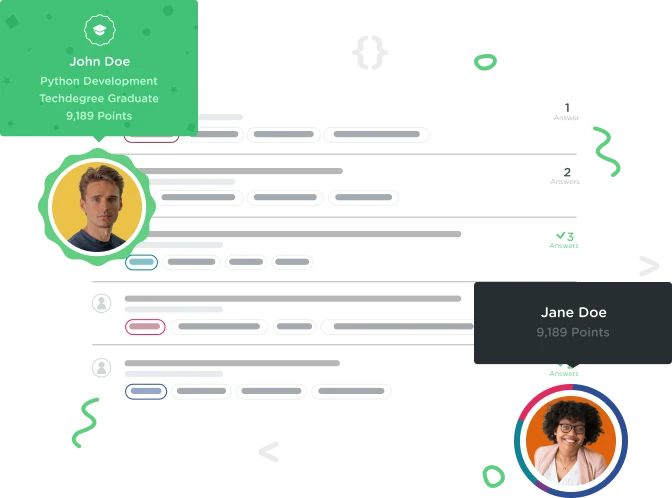
James Moran
7,271 PointsIs it possible to position a button to bottom of screen if within 100vh?
I'm trying to position the button with the id of '#action-buttons' to the bottom of the page. Although this works whether it is visible or not, I would like it to work if it is within 100vh of the window.
function isButtonVisible(button) {
if ($(button).visible()) {
return true;
} else {
return false;
}
}
function positionButton() {
var aButton = '#action-buttons';
$(aButton).css('position', 'static');
if (isButtonVisible(aButton)) {
$(aButton).css('position', 'fixed');
$(aButton).css('bottom', '50px');
$(aButton).width($('#create-advert .main-pages').width());
} else {
$(aButton).css('position', 'static');
$(aButton).css('width', '100%');
}
}
positionButton();
$('#next-button').click(function () {
positionButton();
});
Thanks!!
3 Answers
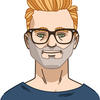
Shane Oliver
19,977 PointsThe window object has several ways to get the height of the client one of them is window.clientheight. You will need to check the window scroll and measure it against the client height to determine whether it is within or outside of bounds
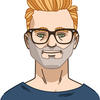
Shane Oliver
19,977 PointsThe window is always 100vh - so the button will always show.
James Moran
7,271 PointsAh okay. Well is there a way to tell if it is within the view of the window?
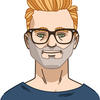
Shane Oliver
19,977 PointsYou can simplify your isButtonVisible function to one line too
return $(button).visible();
James Moran
7,271 PointsOh yeah! Thanks :)
James Moran
7,271 PointsJames Moran
7,271 PointsI followed your advice and solved it by comparing the window height to the position of the button on the page.
Thanks for the help!