Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial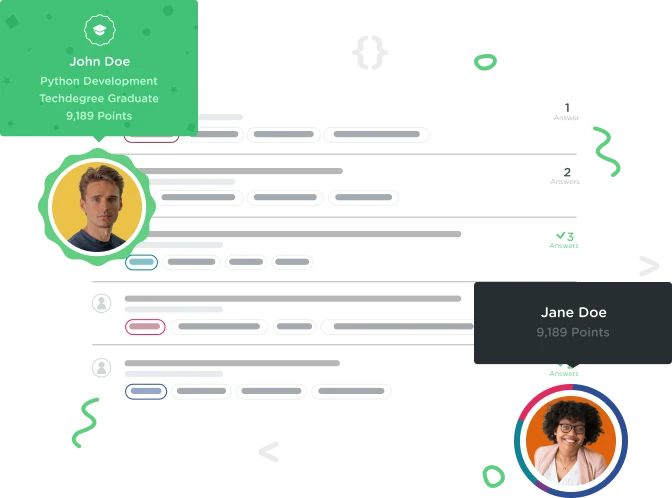

Eduard Edwin
14,569 PointsIs it possible to refactor my code further?
It took me 3 hours to get this code right, and when I finally did, I refactored the code, implementing DRY programming principle. However, I get this nagging feeling that I can refactor my code further. I don't know what it is, I just thought I ask you guys to have a look and give your feedback on it.
My code looks a bit complicated, so I added a few comments for added clarity, other than indentation. Mine differs slightly from Dave's solution; I use functions here for the purpose of reusing this code in a possible future project. Is it alright to have too many functions in a programs? Or does that depends on the size and complexity of the program?
I appreciate any feedback/comment/answer/criticism. :)
Thanks.
var questions = [
["How many states are in the United States?", 50],
["How many continents are there?", 7],
["How many legs does an insect have?", 6]
];
var correctQuestion = []; // Initializing a single array to hold question(s) answered correctly
var wrongQuestion = []; // Initializing a single array to hold question(s) answered wrong
var correct = 0; // Number of correct answer(s)
var wrong = 0; // Number of wrong answer(s)
var answer; // Hold the user's input
// Print the list of question
function printResult(arrayQuestion) {
var listHTML = '<ol>';
for (var i = 0; i < arrayQuestion.length; i += 1) {
listHTML += '<li>' + arrayQuestion[i] + '</li>';
}
listHTML += '</ol>';
print(listHTML);
}
function print(message) {
document.write(message);
}
function quiz(question) {
for (var i = 0; i < question.length; i += 1) {
answer = parseInt(prompt(question[i][0])); // Ask user for input and parse it to an integer
// Check whether the answer is correct/wrong, increment its respective counter by 1
// and store the question by pushing it to the end of the single array
if (answer === question[i][1]) {
correctQuestion.push(question[i][0]);
correct += 1;
} else {
wrongQuestion.push(question[i][0]);
wrong += 1;
}
}
print('You got ' + correct + ' question(s) right.');
// If correct is more than 0, the code inside will be executed; otherwise move to the next if statement
if (correct > 0) {
print('<h2>You got these question(s) correct:</h2>');
printResult(correctQuestion);
}
// This if statement will only be executed if wrong is more than 0
if (wrong > 0) {
print('<h2>You got these question(s) wrong:</h2>');
printResult(wrongQuestion);
}
}
quiz(questions); // Call the function quiz
1 Answer
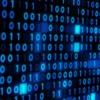
Alexander Davison
65,469 PointsI can see a small refactoring, but it seems like a good idea to add.
Anything other than what I'm seeing right now looks fantastic.
I'm focusing on a small part of your code, so that's why I'm not closing the curly brace for the function.
This is your code:
function quiz(question) {
for (var i = 0; i < question.length; i += 1) {
answer = parseInt(prompt(question[i][0])); // Ask user for input and parse it to an integer
// Check whether the answer is correct/wrong, increment its respective counter by 1
// and store the question by pushing it to the end of the single array
if (answer === question[i][1]) {
correctQuestion.push(question[i][0]);
correct += 1;
} else {
wrongQuestion.push(question[i][0]);
wrong += 1;
}
}
And here's my refactoring added on:
function quiz(question) {
for (var i = 0; i < question.length; i += 1) {
var questionToAsk = question[i][0]; // As you see, I made a new variable to store the question to ask.
answer = parseInt(prompt(question[i][0])); // Ask user for input and parse it to an integer
// Check whether the answer is correct/wrong, increment its respective counter by 1
// and store the question by pushing it to the end of the single array
if (answer === question[i][1]) {
correctQuestion.push(questionToAsk);
correct += 1;
} else {
wrongQuestion.push(questionToAsk);
wrong += 1;
}
}
I know, It's a small refactoring, but it makes the code easier to understand and that is also important in programming
When programming, your code should be easy-to-understand, short, and easy to add additions, features and updates. If you write code this way, it is easier for you to understand and to update, and to debug if there are errors in your code!
The thing is, stick to clean code, but; also don't forget to make your program fast and eay-to-use for the people using the program! If your program takes too long to load (5 seconds usually is maximum), users will leave. Yep, that's right, LEAVE. If your program is too slow, they might think it isn't working.
I hope this helps. ~Alex
Eduard Edwin
14,569 PointsEduard Edwin
14,569 PointsHey Alex,
Thanks for the feedback. Appreciate it bro! :)
Alexander Davison
65,469 PointsAlexander Davison
65,469 PointsNo problem