Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial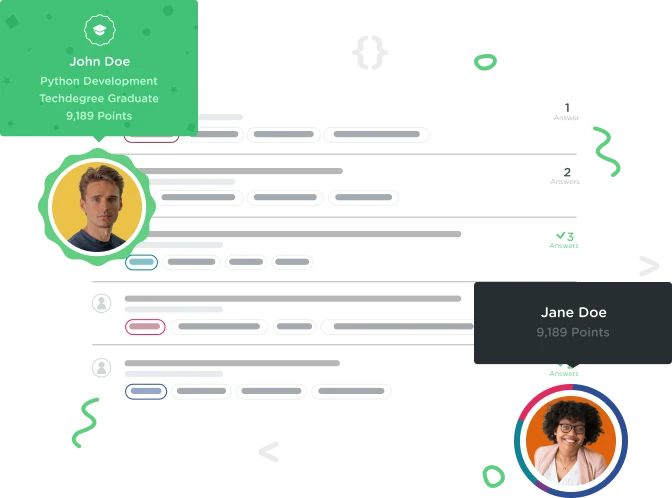

jbong0
6,356 PointsIs it really necessary to store functions into a variable?
ie.. var randNum = randomNumber(6)
rather than just go straight with just doing
var count= 0;
while(count< 10){
randomNumber(6);
counter += 1;
document.write(randomNumber(6) + ' '); /I can even concatenate it to a string
}
I really would like to know the main purpose of this practice.
1 Answer

James Anwyl
Full Stack JavaScript Techdegree Graduate 49,960 PointsHi jbong0,
We are storing the return value of the function, rather than the function itself, in the randNum variable.
The reason we do this is because it is more efficient to call randomNumber() once and store the result in a variable, than call randomNumber() twice.
// Here we call randomNumber twice
randomNumber(6);
counter += 1;
document.write(randomNumber(6) + ' ');
// Here we call randomNumber just once and save the result
var randNum = randomNumber(6)
document.write(randNum + ' ');
counter +=1;
The outcome is the same, but the second example requires 1 less function call. It also has the added advantage that we can re-use the variable as many times as we want, and it's still just 1 function call.
Say it takes 1 second for randomNumber() to finish executing. If we call it 5 times, that's 5 seconds.
document.write(randomNumber(6)); // 1 second
document.write(randomNumber(6)); // 1 second
document.write(randomNumber(6)); // 1 second
document.write(randomNumber(6)); // 1 second
document.write(randomNumber(6)); // 1 second
// Total: 5 Seconds
Whereas if we call it once and then store the value in a variable. We can save ourselves 4 seconds.
var randNum = randomNumber(6); // 1 second
document.write(randNum);
document.write(randNum);
document.write(randNum);
document.write(randNum);
document.write(randNum);
// Total: 1 second
Hope this helps :)
Seth L
6,212 PointsSeth L
6,212 PointsWouldn't your latter two examples depend on if you were expecting to see the same random number 5 times, or a different random number each time? For example, I believe in the former, you'll get a new random number each time the document writes, but in the latter it's the same number printed 5 times.
Is this a correct assertion?