Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial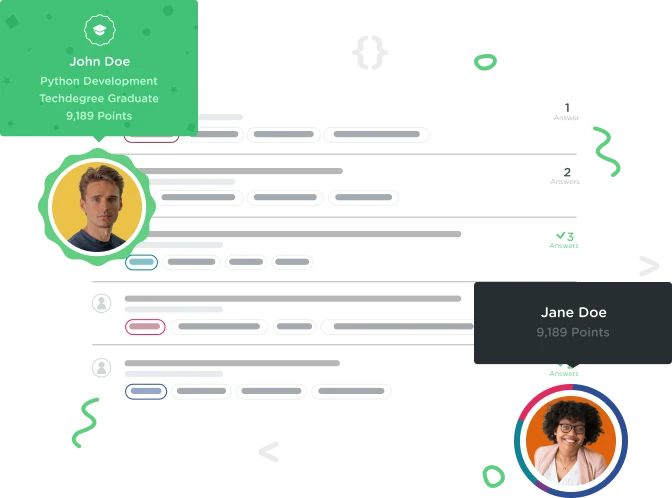
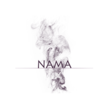
NOUR A ALGHAMDI
Courses Plus Student 5,607 Pointsis it right to do it like this ?
Hello i tried to write the exercise that Mr.Amit asked to do ,and i wrote it as this :
func numdv (#numdiv : Int , #number : Int) -> Bool? {
var numbers = (1...100)
for myQ in numbers {
if numdiv % number == 0 {
return true
} else {
return nil
}
}
if let Answer = numdv(numdiv: 9, number: 4){
println("Divisible")
} else {
println("not Divisible")
}
}
is it right ? Now i think it's right way to write it like this but i don't Know i still have mistakes !
5 Answers
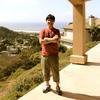
Richard Lu
20,185 PointsHey Nour,
Here's the solution which also guides you through how someone would approach this problem.
// the function takes 2 int parameters
func areNumsDivisible(num1 num1: Int, num2: Int) -> Bool? /* returns an optional bool */ {
// this part, the function is figuring out if the first parameter is divisible by the second
// if a number is divisible by another, then it should have no remainder (hence the modulus operator)
if num1 % num2 == 0 {
// if the number is divisible it returns a true
return true
} else {
// otherwise it returns a nil
return nil
}
}
// calling the function with 2 numbers
let x = areNumsDivisible(num1: 1, num2: 1)
// printing out whether “Divisible” or “Not Divisible”
if x != nil {
print("Divisible")
} else {
print("Not divisible")
}
Hope I've helped. Happy Coding! :)
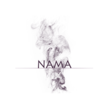
NOUR A ALGHAMDI
Courses Plus Student 5,607 PointsSo the way that you used is the only way to write this code ?
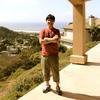
Richard Lu
20,185 PointsHey Nour,
Not exactly, there are other methods of approaching this problem, but they all follow the same guidelines. Keep in mind that sometimes there are multiple solutions to a problem. Here's another way you can do it (slightly a bit more advanced).
func areNumsDivisible(num1 num1: Int, num2: Int) -> Bool? {
return num1 % num2 == 0 ? true : nil
}
print(areNumsDivisible(num1: 1, num2: 1) != nil ? "Divisible" : "Not Divisible")
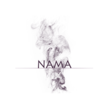
NOUR A ALGHAMDI
Courses Plus Student 5,607 Pointsaha thats my point how to learn those several ways to approaching problems ? how to get this level of learning ?
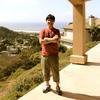
Richard Lu
20,185 PointsWith lots of practice! You'll eventually notice that some things can be placed together like a puzzle.
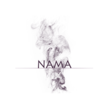
NOUR A ALGHAMDI
Courses Plus Student 5,607 PointsThank you Richard Lu ,
Nour. . .
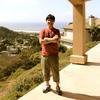
Richard Lu
20,185 PointsMy pleasure Nour! I'm always looking to help :)
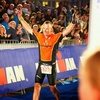
Steve Hunter
57,712 PointsI think there's some additional clarity available here when considering the unwrapping of an optional value. Testing for nil
works in this instance, but the purpose of the optional, and the if let
construct, is lost this way.
I recalled writing a response a while ago for another similar question so thought I'd share that here as it discusses the isDivisible
function, the purpose of optioanl unwrapping and a few other questions that were raised. I think it is relevant to this question, so thought I'd post it here
Hope it adds something for you.
Steve.
Richard Lu
20,185 PointsRichard Lu
20,185 PointsI've fixed up the snippet of code for readability. :)