Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial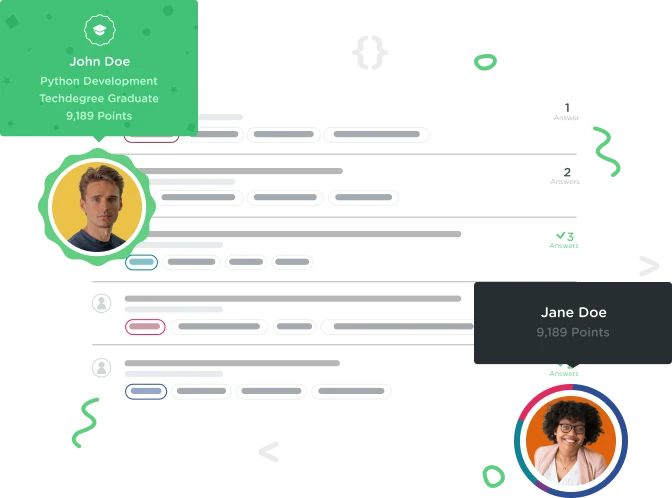
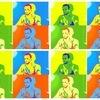
Florian Lindorfer
16,415 PointsIs it the "right" way?
Hi there,
So, my code basically works, but would be nice if one of the pros could do a code review and give me some suggestions/concepts for improvements :)
import datetime
import logging
import random
from questions import Add, Multiply
class Quiz:
questions = []
answers = []
def __init__(self):
for _ in range(10):
# Generate 10 random questions with numbers from 1 to 10
random_num1 = random.randint(1, 10)
random_num2 = random.randint(1, 10)
question_types = [Add(random_num1, random_num2), Multiply(random_num1, random_num2)]
chosen_type = random.choice(question_types)
# Add there questions into self.questions
self.questions.append(chosen_type)
def take_quiz(self):
while True:
# Log the start time
logging.basicConfig(level=logging.DEBUG, filename="quiz.log")
start_time = datetime.datetime.now()
elapsed_time = datetime.timedelta()
logging.debug("Quiz started: {}".format(start_time))
# Ask all of the questions
for question in self.questions:
elapsed_time += self.ask(question)
# Log the end time
end_time = datetime.datetime.now()
logging.debug("Quiz ended: {}".format(end_time))
# Show a summary
self.summary()
print("Elapsed time: {} seconds!".format(elapsed_time.seconds))
break
def ask(self, question):
# Log the start time
start_time = datetime.datetime.now()
# Capture the answer
answer = input("{} = ".format(question.text))
# Check the answer
while type(answer) != int:
try:
answer = int(answer)
except ValueError:
print("This is not a valid number. Please try again!")
answer = input("{} = ".format(question.text))
# If the answer is right, send back True
# Otherwise send back False
if answer == question.answer:
self.answers.append(True)
logging.debug("Answer correct")
else:
self.answers.append(False)
logging.debug("Answer wrong")
# Log the end time
end_time = datetime.datetime.now()
# Send back the elapsed time
elapsed_time = end_time - start_time
logging.debug("Question was answered in {}".format(elapsed_time))
return elapsed_time
def summary(self):
# How many you got right and the total # of questions, 5/10
correct_answers = self.answers.count(True)
print("You answered {}/10 questions correctly!".format(correct_answers))
# Print the total time for the quiz: 30 seconds!
# Testing time
quiz = Quiz()
quiz.take_quiz()