Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial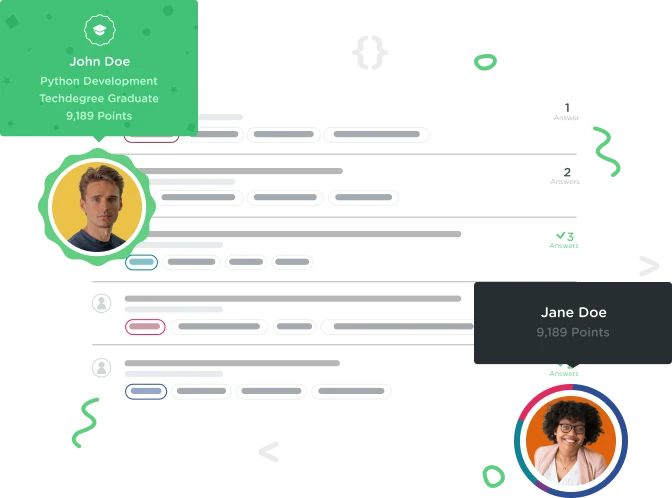
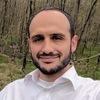
John Pierce
Courses Plus Student 5,108 Pointsis let a bad idea in for loops which will iterate many times?
Since let has block level scoping, every iteration through the loop creates in new instance of the variable. Example:
for(let i=0; i<1000000; i++)
{ //do something a million times }
would create in memory one million instances of the variable i.
If block level scoping wasn't needed inside the for loop, then
for(var i=0; i<1000000; i++)
{ //do something a million times }
would create in memory only one instance of the variable i and just increment its value as the loop progressed. Since javascript uses 64-bit variables, using var in this instance would save A LOT of memory, 8MB! In short - seems to me that there are certainly instances where using var is a better choice than let.
2 Answers
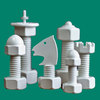
Steven Parker
241,809 PointsUsing "let" in a loop should not require additional memory.
In both of your examples there should only be one variable "i". The difference is that when you use "let", the variable is only available inside the loop. But if you use "var" it is available outside the loop as well.
Perhaps you were thinking about what happens if you declare a function inside the loop which uses it. In that case, it's important for the function to have it's own copy to work correctly. But for the loop itself you should only have one variable.

Taylor Bryant
7,395 PointsI read this and since I am working with geoJSON files that each contain 1400 features I decided to give this a shot and test it out. I at first had not used let at all and had the belief that a variable created without var was global scoped and one created with var was local and block scoped. When I went through a changed all the variables in the object I am using to parse the 2 geojson files together I noticed a drop in 20kb of size. Then I went and changed all the loop iterators to let and noticed an increase of 2kb in size. So it does seem that let does increase the memory consumption of the loop. This might be very useful depending on if you are using lots of loops to parse big data as a 2x increase of the size of these files will not mean that those variables will consume 2x more, they will raise in an upward curve based on the amount of loops used to parse the data.
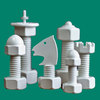
Steven Parker
241,809 PointsIt might increase memory usage, based on what is happening within the loop. Your loops apparently do something that causes the iterators to be retained.
John Pierce
Courses Plus Student 5,108 PointsJohn Pierce
Courses Plus Student 5,108 Pointsthanks for the answer, but you missed what I am saying. Yes, there is only one "i" variable, however, each iteration through the for loop seems to create a new instance of "i" which is scoped to the current iteration of the for loop. Look at the following code and tell me if I'm wrong:
in this case, when a button is clicked, regardless of which one, the alert will always say "Button 3 Pressed". However, if you use "let i=0" then each button will receive its own block scoped copy of "i" and the alert will produce the correct output. So, it certainly appears that there are four different instances of "i" floating around in memory, correct?
Steven Parker
241,809 PointsSteven Parker
241,809 PointsThat's exactly what I was talking about when I said, "Perhaps you were thinking about what happens if you declare a function inside the loop..." The additional variable is within the anonymous event handler function that is created. So it's not the loop itself, but those four functions that each have their own "i", and they need it to function correctly. The memory taken by the variables is small compared to all those separate copies of the function.
For memory conservation in a situation like this, I would recommend creating a single delegated event handler outside the loop that handles the events from all of the buttons.