Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial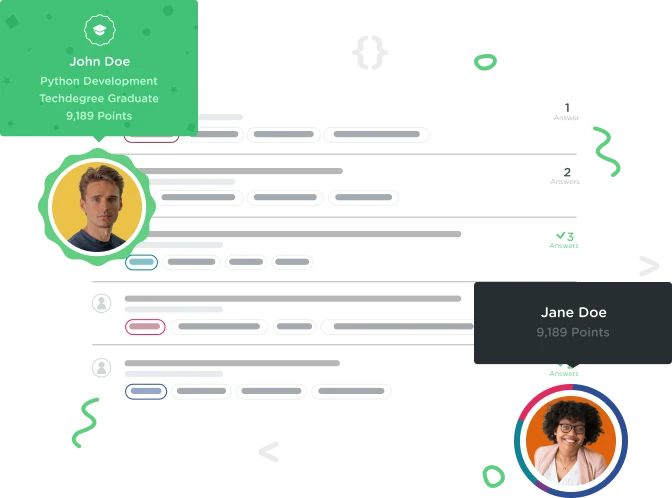
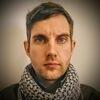
Alex Hort-Francis
17,074 PointsIs 'let' compulsory when declaring 'i' in the for loop?
I've noticed that the following code will work in the browser (Chrome):
for (i=0; i<10; i++) {
console.log(i);
}
The output is:
0
1
2
3
4
5
6
7
8
9
With that in mind, I am interested whether it is technically compulsory to use let
to declare i
in the for loop syntax.
Does this have something to do with 'strict mode' and avoiding potentially buggy code?
Thanks! :)
4 Answers
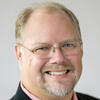
Jason Larson
8,361 PointsYou are correct. In JavaScript, by default you are not required to declare variables before using them, so the following statements are basically the same (other than scope):
i = 0;
let i = 0;
var i = 0;
The problems come in when strict mode is enabled or using other frameworks like React that require strict mode. Additionally, you can run into some bugs that are harder to troubleshoot when you don't declare the variable type because they will become global variables by default.
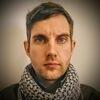
Alex Hort-Francis
17,074 PointsI'm back here again, I Googled my own question haha
I think the takeaway is just use let
in the for loop, for best practice -- at least that's what I'll be doing from now on..
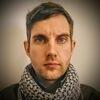
Alex Hort-Francis
17,074 PointsThat is very interesting.
I suppose i
in this case would be automatically initialised as a let
? Or would it be a var
?
It sounds like it would be best to always declare i
properly in a for loop then (using let
).
Otherwise, if i
is being automatically created as a global variable, would that mean that any other javascript run on that page would be able to access it? So if another i
is used in a separate script it could cause collision problems?
Thanks!
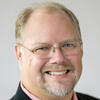
Jason Larson
8,361 Pointsi would be automatically declared as a var, since var is global. The let declaration is block-scoped, so if you declare it in a for loop, it will go out of scope once you exit the loop. Honestly, I'm not sure about collisions from another script, as it may depend on how the script is accessed, but certainly any other parts of the same script could read or write to the variable.

Alex Hort-Francis
12,924 PointsUpdate for 2023
According to our good friend GPT-4:
In JavaScript, it's not compulsory to use let
when declaring a loop variable like i
in a for
loop, but it's a recommended best practice for various reasons. Here's a deeper dive into your question:
-
Without
let
in Non-strict Mode: If you don't uselet
(orvar
) and you're not in strict mode, then the variablei
will be implicitly declared in the global scope. This is problematic because it can lead to unexpected bugs or overwrite other variables with the same name in the global scope.
for (i=0; i<10; i++) {
console.log(i);
}
console.log(window.i); // 10 (in browsers)
-
Without
let
in Strict Mode: If you enable strict mode with'use strict';
, the above code will throw an error because strict mode doesn't allow implicit global variable declarations.
'use strict';
for (i=0; i<10; i++) {
console.log(i);
}
// Uncaught ReferenceError: i is not defined
-
Using
let
: When you uselet
to declare the loop variable, it ensures the variable only exists in the loop's block scope. This is the recommended approach because it avoids polluting the global scope or the surrounding function scope.
for (let i=0; i<10; i++) {
console.log(i);
}
console.log(typeof i); // "undefined"
Historical Context with
var
: Beforelet
was introduced in ES6 (ES2015), the only option for variable declarations wasvar
. Unlikelet
,var
has function scope instead of block scope. This could lead to subtle bugs and was one of the reasonslet
(with its block scoping) was introduced.Strict Mode: Enabling strict mode (
'use strict';
) helps catch common coding mistakes. In the context of your question, strict mode will prevent implicit global declarations, thereby forcing you to declare your loop variable explicitly.
In summary, while you might be able to get away without using let
in some contexts, it's a good habit to always declare loop variables with let
to avoid potential issues and make your code more maintainable.