Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial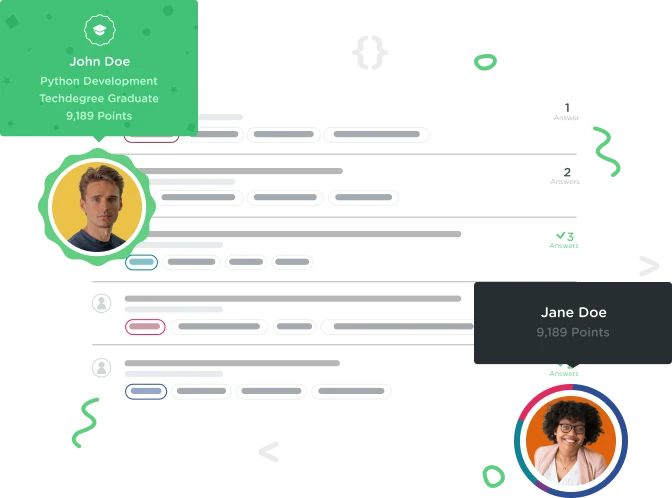

john sec
2,304 PointsIs my code too long? Can you give me an advice on how to shorten this code? or just any kind of advice?
"""
Run the script to start using it
Put new things into the list, one at a time
Enter the word DONE - in all caps - to quit the program
And, once I quit, I want the app to show me everything that's on my list
to_do_list = []
def additional_item(): """ Adds an item to the list """ while True: additional = input("Add an item into your list: ") if additional == "DONE": break to_do_list.append(additional) return to_do_list
def remove_item(): """ Remove an item to the list """ removed = input("Choose an item to remove: " + ", ".join(to_do_list) + ": ") to_do_list.remove(removed) return to_do_list
def menu(): """ Shows the menu of the app""" print( """ 1 - View the list 2 - Add an item "Type DONE - ALL caps when finish adding." 3 - Remove an item 4 - Exit """ )
def question(): while True: try: user = int(input("Choose a number 1 - 4: ")) except ValueError: print("Not a valid choice try again.") if user > 4 or user <= 0: print("Not a valid choice, Try again.") else: break return user
def number_1(): if not to_do_list: print("Your list is empty. Add an item first.\n") else: for i in to_do_list: print("\t" + "- " + i)
def shopping_to_do_list(): menu() answer = 0 while answer != 4: answer = question() if answer == 1: number_1() elif answer == 2: additional_item() elif answer == 3: remove_item() else: print("Thank You!\n")
if not to_do_list:
print("Your list is empty.")
else:
print("Your shooping to do list.\n")
for i in to_do_list:
print("\t" + "- " + i)
shopping_to_do_list() """
1 Answer
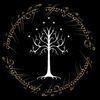
jacinator
11,936 PointsNot sure how much shorter this is, but it should perform the same things.
class ListManager:
todo_list = []
answer = None
menu = {
'1': "View the list",
'2': "Add an item\nType DONE - ALL caps when finish adding.",
'3': "Remove an item",
'4': "Exit".
}
def add_item(self):
add_item = input("Add an item into your list: ")
self.todo_list.append(add_item)
def remove_item(self):
print(", ".join(self.todo_list))
remove_item = input("Choose an item to remove: ")
try:
self.todo_list.remove(remove_item)
except ValueError:
pass
def print_list(self):
if not to_do_list:
print("Your list is empty. Add an item first.\n")
else:
[print("\t- {}".format(item) for item in self.todo_list]
def print_menu(self):
[print("{} - {}".format(shortcut, command) for shortcut, command in self.menu.items()]
def question(self):
try:
self.answer= int(input("Choose a number 1 - 4: "))
except ValueError:
self.answer = None
def run(self):
self.print_menu()
while self.answer != 4:
self.question()
if self.answer == 1:
self.print_list()
elif self.answer == 2:
self.add_item()
elif self.answer == 3:
self.remove_item()
else:
print("Not a valid choice, Try again.")
self.print_list()
ListManager().run()

john sec
2,304 Points@jacinator Good day. how can I make my code look like that sir?

john sec
2,304 Points@jacinator Thank you sir..
jacinator
11,936 Pointsjacinator
11,936 PointsJust putting your code into some markup before trying anything with it.