Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial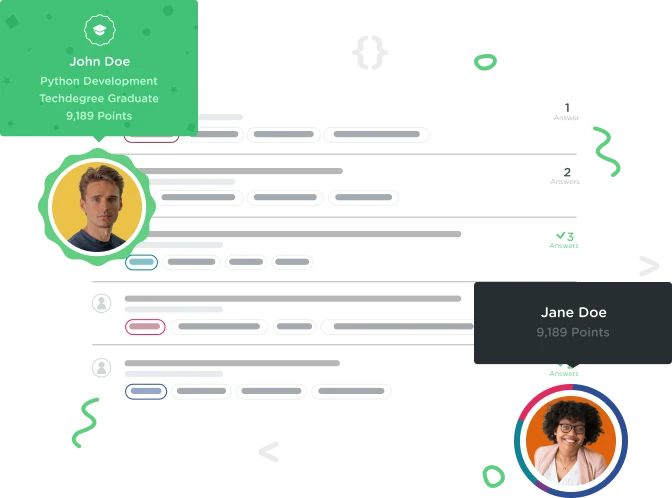

ori
2,307 PointsIs my solution also valid? if ( isNaN(lower) === true | isNaN(upper) === true )
Why is the conditional statement written here as:
if ( isNaN(lower) | isNaN(upper)
And not:
if ( isNaN(lower) === true | isNaN(upper) === true )
I thought you had to specify the condition inside on each side of the ||.
My solution:
function getRandomNumber( lower,upper ) {
if ( isNaN(lower) === true | isNaN(upper) === true ) {
throw new Error('You did not enter a valid number');
} else {
return Math.floor(Math.random() * (upper - lower + 1)) + lower + 1;
}
}
2 Answers
Jesse James
3,020 PointsTo expand upon what Chris Upjohn wrote, I wanted to explain the reason why you don't need to have the "=== true" portion.
As you may remember from an earlier video, you can't have something like the following: if ( userFirstName === 'Bob' || 'Diane')
While "userFirstName === 'Bob'" evaluates to a value true or false value, "diane" by itself is just a string. The conditional doesn't automatically apply the "userFirstName ===" from the first part of the OR statement.
In this case, the functions "isNaN(lower)" and "isNaN(upper)" evaluate the argument passed to the function (lower and upper, respectively) and return a true/false value. So, spelled out, what you're getting is: "Is lower not a number?" || "Is upper not a number". The functions themselves both return a true/false value.
How does that all tie in?
"If" conditionals are looking to see if the argument passed evalutes to true or false. If the arguments ultimately equate to True, it processes the rest of the code contained within its { }. If the arguments end up False, it does nothing or executes the code in the Else conditional directly after it. Since the isNaN(lower) and isNaN(upper) return a true/false value and the "If" conditional is already checking for a true value, there's no need to check again inside the If statement arguments.
Your method of rechecking the True/False would end up with the same result as the recommended solution, but is unnecessary.

Chris Shaw
26,676 PointsHi ,
The operator you have aka the pipe |
is a bitwise operator which doesn't behave the same way as the OR operator, see the below for a quick description for this bitwise operator.
Returns a one in each bit position for which the corresponding bits of either or both operands are ones.
Also just to clarify the evaluation you have === true
isn't required as isNaN
will only ever return true
or false
therefore we can omit this.
if (isNaN(lower) || isNaN(upper))
Hope that helps.
ori
2,307 Pointsori
2,307 PointsThanks a lot Jesse for taking the time to explain, really appreciate it.