Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial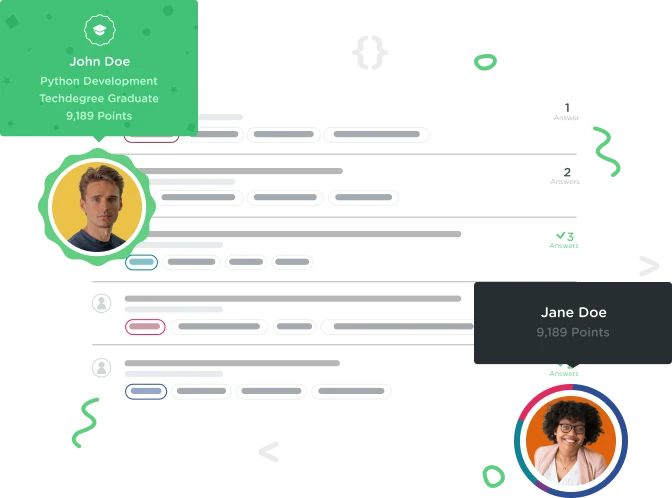

tomasliashuk
8,014 PointsIs my solution worth it? A question about variables and embedded functions
Hello, here is my solution for the challenge. The primary questions are:
- Where should I put variables, if I know they will only be used inside the function? Such as "userAnswer" variable in this case (I know, that next challenge wants me to print them out, but let us assume I don't have to).
- Is it possible and worth to call a function inside a function in this case? Maybe it will take more computing power and I should stick to other method?
Thank you in advance!
Here is the code:
var correctAnswers = 0;
var questions = [
["Who is mr Putin?", "president"],
["How much the time costs?", "much"],
["What do I like?", "javascript"]
]
function print(message) {
document.write(message);
}
function askingQuestions ( parameter ) {
for ( var i = 0; i < parameter.length ; i += 1 ) {
var userAnswer = prompt( parameter[i][0]);
if ( userAnswer.toLowerCase() === parameter[i][1]) {
correctAnswers += 1;
}
}
print(correctAnswers);
}
askingQuestions(questions);
2 Answers
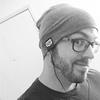
Jesse James
6,079 PointsHello! To help answer your questions:
The general idea from what I've personally seen, in regards to variables, is to place them where they will be used/where they are needed. In the case of "useranswer", since you only need it for the askingquestions function, it wouldn't need to be available for usage in other functions. However a variable like correctAnswers could easily be used/referenced by many methods, so its best to have it in a scope where it's available.
Calling functions within other functions is definitely possible! I find that the best times to do this are when you notice that a function is doing multiple things. If you have a function that is asking questions, evaluating the answer, and then scoring the result for the user, you can likely break that up into several different functions so that if you need to make a change to a specific part of the logic later you can just focus on that specific function rather than having to find it within a jumble of code doing many different things.
That may be a bit hard to follow, let me know if you'd like me to expand upon any thing!

Iain Simmons
Treehouse Moderator 32,305 PointsI'd also suggest changing the name of the parameter
parameter to something that better represents what it will hold.
You can even reuse questions
, as it will only refer to the parameter inside the function and not the variable outside the function.
Otherwise something like questionList
would be suitable.
In my solution I had a function for asking a single question and returning a true
or false
depending on whether the user got it right. My loop was on the outside, and called the function each time through:
var correct = 0;
var minResponseLength = 5;
var questions = [
["What is the largest living land animal?", "african elephant"],
["What is the largest living reptile?", "saltwater crocodile"],
["What is the largest living fish?", "whale shark"],
["What is the largest living bird?", "common ostrich"],
["What is the tallest living animal?", "giraffe"]
];
function print(message) {
document.write(message);
}
// a function to ask the question and return true if answered correctly
function quizQuestion(questions, questionNumber) {
// get the user's response to the question being asked, convert to lowercase
var response = prompt(questions[questionNumber][0]).toLowerCase();
/* return true if the user's response exists within
or is equal to the actual answer
AND the response is greater than or equal to the min characters
(so they can't cheat with a random letter or two)
- this allows for responses that aren't the full string in the answer */
return (
questions[questionNumber][1].indexOf(response) >= 0
&&
response.length >= minResponseLength
)
}
for (var i=0; i<questions.length; i++) {
if (quizQuestion(questions, i)) {
correct++;
}
}
print('<p>Out of ' + questions.length + ' questions, you got ' + correct + ' correct and ' + (questions.length - correct) + ' incorrect.</p>');
john larson
16,594 Pointsjohn larson
16,594 PointsΠΏΡΠΈΠ²Π΅Ρ Comrad, I am in the middle of this challenge as well. I like your code. it's very clean and to the point. I copy and pasted it to my editor to see if it worked, of course you know it did. I'm going to examine how you did so cleanly what seems to be potentially messy programming