Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial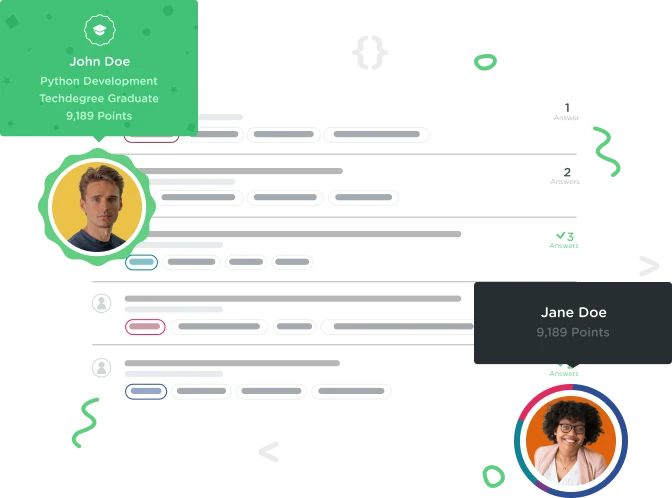

Zailey Walls
5,427 PointsIs my understanding correct about prototypal inheritance?
// product constructor
var Product = function(name, category, price, images, description) {
this.name = name;
this.category = category;
this.price = price;
this.images = [];
this.description = description;
this.on_sale = false;
}
// a function that sets the 'on_sale' property to true
Product.prototype.place_on_sale = function() {
this.on_sale = true;
}
// creating a constructor that inherits from the Product constructor
var Shoe = function(sizes, colors) {
Product.call(this, name, category, price, images, description);
this.sizes = [];
this.colors = [];
}
// my undestanding of the code below is that it copies and pastes the prototypes (methods) into the 'Shoe' concstructor
Shoe.prototype = Object.create(Product.prototype);
I not sure why 'this' is the first argument when we call the Product constructor...
1 Answer
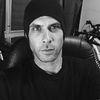
jack AM
2,618 PointsI'll try to take a stab at *this,
The "this" keyword sets the context of what object you're referring to when your code runs. Lets take a snapshot, just for a second, down the road when you have created say, 15, different Shoe objects being shown on your website. Every Shoe object would ideally have at least one thing/attribute that is different (name, color, size etc...), from the rest of the Shoes. After all, each Shoe would be shown individually for a reason no?
So in this regard the "this" keyword prepended to your attribute names (when the object gets created) tells the interpreter that you're referring to THAT specific Shoe, and NOT referring to ALL of the Shoe objects in your awesome factory, when these variables are getting assigned their values.
And with the "call" method that you're using to inherit the Products properties, JavaScript just wants to know in what context you're referring to as it allows your Shoe object to inherit the properties of the Product object, just that one shoe? Or all of them. If it's just that one shoe, then pass in the "this" keyword. Again this is because you want to set the properties of each object specifically for THAT object, and not blanket the color green, for example, to all shoes in your factory. If you want, take out the keyword "this" from the contructor, and see how the properties of the each Shoe will be affected. It might give you a better idea of how context is used with JavaScript. Just an idea.
Also, https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/Function/call is just a quick reference for the call method, and states that "this" is the first argument that should be provided.