Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial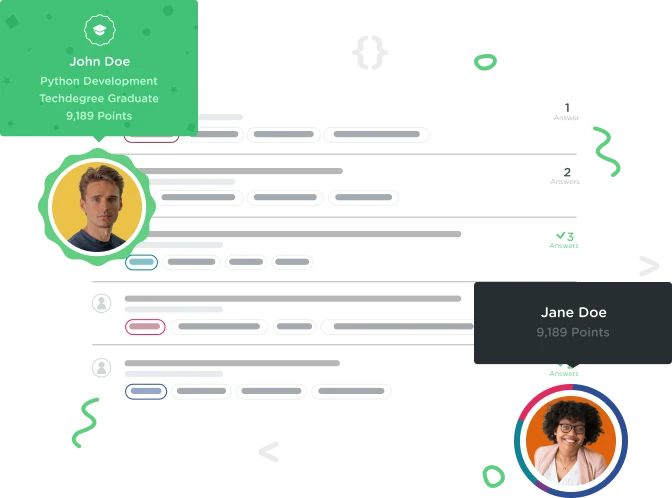

Lukasz Walczak
6,620 PointsIs my understanding of down / upcasting correct?
Hi, guys!
Since that video I'm lost. Some times I think I got it, but most of the times I do not :) I tried to watch some tutorials, but It seems that I cannot grasp this concept completely.
Can someone pls point me in the right direction?
Many thanks!
//creating new object called treet of type Treet
Treet treet = new Treet("Craig Dennis", "Example text", new Date());
//puting Treet object in to the obj object of type Object
//all treet methods and variables are not accessible
//WAS THAT UPCASTING?
Object obj = treet;
//What are we actuallly doing here?
//Are we downcasting?
//What it actually means?
//Is this treet or object
Treet another = (Treet)obj;
2 Answers
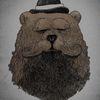
Mihai Craciun
13,520 Points//creating new object called treet of type Treet
Treet treet = new Treet("Craig Dennis", "Example text", new Date());
//This is Upcasting yes.
//Because Object is a superclass for Treet so we could assign it to another variable like below.
Object obj = treet;
//This is Downcasting.
//It sais to compiler to treat the obj Object as a Treet Object so you more specified
Treet another = (Treet) obj;
Object obj = treet;
Treet treet = new Treet("Craig Dennis", "Example text", new Date());
//Because of the type chain treet have all the methods both Treet and Object types have
//So both statements below are correct
treet.getName(); //this is an Treet method
treet.equals( anotherTreet ); //this is a Object method
//But if you would use the obj variable instead one of the two would generate an error
obj.getName(); //this is incorrect because Object type does not have a getName() method;
obj.equals( anotherTreet ); //this is correct because is obj is Object type
//but if we cast the obj variable as a treet we can use both methods again
Treet another = (Treet) obj;
another.getName(); //correct
another.equals( anotherTreet ); //correct
Now maybe you wonder why do you need Type casting. Well type casting is very useful when you don't know the object type. There are some method that will just return an Object and you know the type that it should be but you can't use any of it's methods because it is casted as an Object. Let me give you an example of my own.
//This is a part of o chat that sends Strings from a Client to a Servers.
//I will not give many details but what is important
Object obj = socket.readObject();
//I know that the client send a String variable but I have to save it as a Object first now because
//the readObject() method returns an object. To utilize it as a String i have to downcast it like below:
String message = (String) obj;
System.out.println(message);
I hope you understand now how type casting works

Lukasz Walczak
6,620 PointsThanks a bunch! Again!
Lukasz Walczak
6,620 PointsLukasz Walczak
6,620 PointsMihai Craciun, I salute you! Many thanks for taking time to answer!
Just to make sure:
Hopefully you got some more time ;)
Mihai Craciun
13,520 PointsMihai Craciun
13,520 PointsYes you got it! Because Java is an object-oriented programming language almost everything can be upcasted in an Object type. But Downcasting is not always possible. For example let's say you have another Object named CastObj:
I hope this information helped you :)