Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial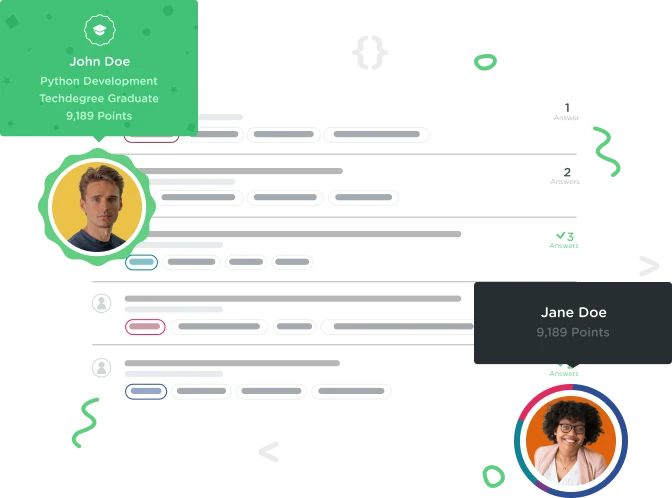
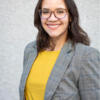
Katiuska Alicea de Leon
10,341 Points"Is not a function" error
I'm trying to recreate to this very simple example and I really don't see where I'm going wrong. I tried doing this example but I couldn't on my own. After typing and typing, checking every line, I opted to just copy from the exercise file the snippet and then it worked, so I'm a moron.
I tried then doing the className example with a website I'm working on. It should have worked the same but it doesn't and I don't know why. Can someone tell me what I'm doing wrong? Why is Guil's a function and not mine?
Thanks
// Guil's code
var myHeading = document.getElementById('myHeading');
myHeading.addEventListener('click', () => { myHeading.style.backgroundColor = 'pink'; });
// My code
var myList = document.getElementsByClassName('nav-li');
myList.addEventListener('click', () => { myList.style.backgroundColor = 'pink'; });
7 Answers

KRIS NIKOLAISEN
54,971 PointsUnlike getElementById()
, getElementsByClassName()
returns a collection. So you will want to assign an individual item from the collection.
var myList = document.getElementsByClassName('nav-li')[0];
Note the [0] index at the end. This is provided you have an element with a class name = 'nav-li'
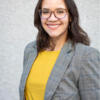
Katiuska Alicea de Leon
10,341 PointsOhhh, you beautiful genius!! Thank you so much!!! I can't believe I'm going to have to rewatch the whole DOM course again. I took a few weeks off and I've forgotten EVERYTHING!!
Again, thanks for your help!
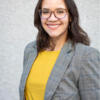
Katiuska Alicea de Leon
10,341 PointsYou know, selecting an index works if I want to select only one item in the collection but I tried creating a loop so that when I click on any of the elements belonging to the 'nav-li' class would change color when clocked on but again I'm getting the not a function error. Here's my code. Can you help me again?
var myList = document.getElementsByClassName('nav-li'); myList.addEventListener('click', () => {
for(i = 0; i < myList.length; i += 1) {
myList[i].style.backgroundColor = 'pink';
}
});

KRIS NIKOLAISEN
54,971 PointsYou are trying to use addEventListener
with collection myList
. Instead you would need to loop through the collection and use addEventListener
with each element
var myList = document.getElementsByClassName('nav-li');
for(i = 0; i < myList.length; i += 1) {
myList[i].addEventListener('click', function() {
this.style.backgroundColor = 'pink';
});
};
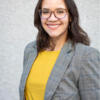
Katiuska Alicea de Leon
10,341 PointsI see what I did wrong: I started with the event listener when instead I should have started the loop and put the event listener function INSIDE the loop. Also, I never use 'this', as I'm not sure how to use it and it wasn't on this course. I first saw it in a later Object Oriented JavaScript course.
I'm using the code with 'this' instead of "myList.style.backgroundColor = ' pink';" because using myList is simply not working. It says cannot set property of undefined. Why is that so? Guil never used 'this' on this course, not to my recollection.
Thank you, Kris!

KRIS NIKOLAISEN
54,971 PointsHere is an explanation of why you see undefined. There is also a recommendation to not use the loop at all and instead use event delegation. Then the code might look like this:
document.body.addEventListener('click',function(e){
if (e.target.className == 'nav-li'){
e.target.style.backgroundColor = 'pink';
}
});
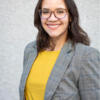
Katiuska Alicea de Leon
10,341 PointsThe bubbling? Thank you, Kris. I will check out the link. You've been amazing!