Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial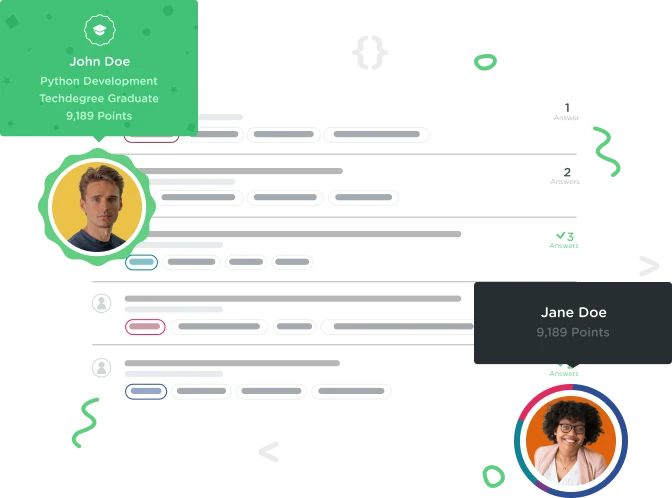

Junior Aidee
Front End Web Development Techdegree Graduate 14,657 PointsIs the below solution correct as well? It seems to work fine for me.
// Collect input from a user const lowUserInput = parseInt ( prompt("Please enter your first number.") ); const highUserInput = parseInt ( prompt("Please enter your second number.") );
// Convert the input to a number
if(lowUserInput && highUserInput) { // Use Math.random() and the user's number to generate a random number const randomNumber = Math.floor ( Math.random() * highUserInput ) + lowUserInput;
// Create a message displaying the random number
alert(${randomNumber} is between ${lowUserInput} and ${highUserInput}
);
// Create a message displaying the random number
} else {
console.log(Please enter a valid number.
)
}
2 Answers
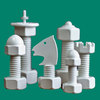
Steven Parker
231,268 PointsAs already pointed out, the formula to create a number within a range is not correct.
Finding issues like this often requires be clever about the test scenarios, as simple examples might seem to be working. For this case, try using this formula to get a few samples of numbers between 90 and 99.
Also, using the && operator by itself isn't an effective way to test numbers for validity (the "isNaN" function can be useful). Try testing the current code by asking for a number between 0 and 9.

Junior Aidee
Front End Web Development Techdegree Graduate 14,657 PointsThank you guys!
Tai Jun Jie
Full Stack JavaScript Techdegree Graduate 23,907 PointsTai Jun Jie
Full Stack JavaScript Techdegree Graduate 23,907 PointsHey, the solution below is incorrect.
randomNumber = Math.floor ( Math.random() * highUserInput ) + lowUserInput;
there is just 1 problem with this code. This code above would return a max number of (highest input + lowest input) instead of just the highest input. In order to fix this, you can see the code below
randomNumber = Math.floor ( Math.random() * (highUserInput-lowestUserInput + 1) ) + lowUserInput;
As you can see, you need to minus the lowUserInput from ( Math.random() * (highUserInput-lowUserInput + 1) . You add 1 in order to include the lowUserInput and the highUserInput in the range.
For more info, you can use this link. https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/Math/random