Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial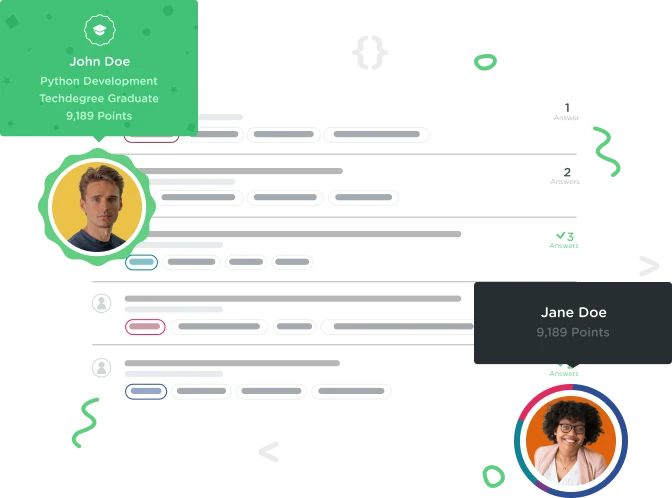

elizabethkari
15,539 PointsIs the code posted somewhere? It's not in the downloads. I've made a type while following along and want to compare.
I'm sure it all occurred during the cutting/pasting in the routes. I've tried re-watching and pausing the video, and linting, but I can't find it. As a result I can't start the server. It would be helpful to have something to compare it to.
2 Answers

Dylan Gaffney
Courses Plus Student 4,781 PointsHere you go! I copied my code from the next section which includes some error handling on the /vote-:dir route. Make sure you check that you have req and res in the proper position for your route callbacks. I mixed them up and spent 20 minutes trying to figure out why I was getting a Type error on 'dir'.
App.js
var express = require('express')
var app = express()
var router= require('./routes')
var jsonParser = require('body-parser').json
var logger = require('morgan')
app.use(logger('dev'))
app.use(jsonParser())
app.use('/questions', router)
// catch 404 and forward to error handler
app.use(function(req, res, next){
var err = new Error('Not Found')
err.status = 404
next(err);
});
// Error Handler
app.use(function(err, req, res, next){
res.status(err.status || 500);
res.json({
error: {
message: err.message
}
})
});
var port = process.env.PORT || 3000;
app.listen(port);
console.log('The server is running on port', port);
routes.js
'use strict';
var express = require('express')
var router = express.Router();
//Get Questions from /questions
router.get('/', function(req, res){
res.json({response: 'You sent me a GET request'});
});
//Post data to questions
router.post('/', function(req, res){
res.json({
response: 'You sent me a POST request containing: ',
body: req.body
});
});
//get a particular question based on id
router.get('/:qID', function(req, res){
res.json({
response: 'You sent me a GET request for ID ' + req.params.uID
});
});
//POST New Answer for a Question
router.post('/:qID/answers/', function(req, res){
res.json({
response: 'You sent me a GET request for user ' + req.params.uID,
userID: req.params.qID,
body: req.body
});
});
//PUT Update a question with a new answer (or update an existing answer)
router.put('/:qID/answers/:aID', function(req, res){
res.json({
response: 'You sent me a PUT request for answer ' + req.params.aid,
questionID: req.params.qID,
answerID: req.params.aID,
body: req.body
});
});
//DELETE a specific answer for a question
router.delete('/:qID/answers/:aID', function(req, res){
res.json({
response: 'You sent me a DELETE request for answer' + req.params.aID,
questionID: req.params.qID,
answerID: req.params.aID
});
});
//POST an up or down vote for a particular answer
router.post('/:qID/answers/:aID/vote-:dir', function(req, res, next) {
if(req.params.dir.search(/^up|down$/) === -1){
var err = new Error('Parameter Not Found');
err.status = 404;
next(err);
} else {
next();
}
}, function(req, res){
res.json({
response: 'You sent me a POST request to /vote-' + req.params.dir,
userID: req.params.qID,
accountID: req.params.aID,
vote: req.params.dir
});
});
module.exports = router;
Marie Nipper
22,638 PointsMarie Nipper
22,638 PointsI've messed stuff like that up plenty of times. Can you paste your routes.js and app.js code?