Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial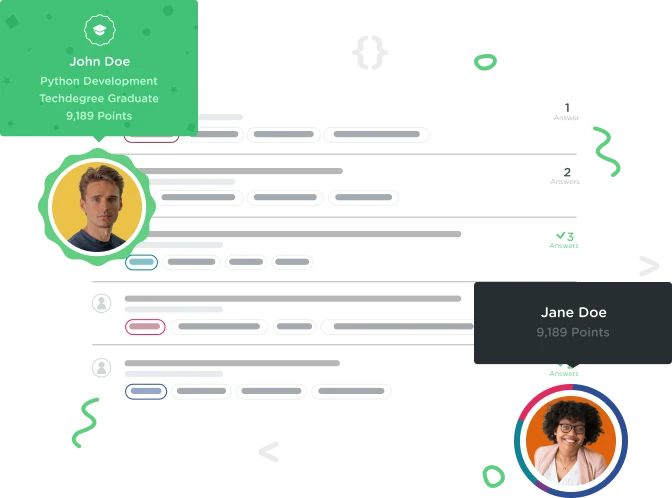

Britt Green
2,611 PointsIs the Icon enum() portion superfluous?
If our Dark Sky API sends us the name of the icon, such as "clear-day", why do we convert that to .ClearDay in our enum, instead of just doing a switch statement that says case clear-day = "clear-day.png"?
2 Answers

Enrique Munguía
14,311 PointsYeah you are right, you can write a switch statement checking the string you receive and it will work. In this case an enum is used to encapsulate all expected cases, this helps to have a better documented code stating which values are legal to use.

Karl Metum
3,447 PointsAlso, we do not need the switch statement at all!
We could simple rewrite the Icon enum like this:
enum Icon: String {
case ClearDay = "clear-day"
case ClearNight = "clear-night"
case Rain = "rain"
case Snow = "snow"
case Sleet = "sleet"
case Wind = "wind"
case Fog = "fog"
case Cloudy = "cloudy"
case PartlyCloudyDay = "partly-cloudy-day"
case PartlyCloudyNight = "partly-cloudy-night"
func toImage() -> UIImage {
return UIImage(named: "\(self).png")
}
}
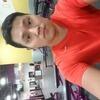
Tommy Choe
38,156 PointsThanks! You answered my question.
Edit: Just a heads-up. So the images for a cloudy day and cloudy night are named "cloudy-day.png" and "cloudy-night.png" NOT as "partly-cloudy-day.png" and "partly-cloudy-night.png".
I decided not to change the image name and handle it with conditionals instead.
func toImage() -> UIImage?{
print("\(self.rawValue).png")
if self == .PartlyCloudyDay {
return UIImage(named: "cloudy-day.png")
} else if self == .PartlyCloudyNight {
return UIImage(named: "cloudy-night.png")
} else {
return UIImage(named: "\(self)")
}
}
Anyways happy coding!
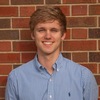
Grayson Webster
11,528 PointsMake sure to change "self" to "self.rawValue" if you use this method.

KB Li
Courses Plus Student 625 PointsHi Karl, this is a very good practice.
However, by doing string interpolation, we have to make sure they(images) are all in the same format PNG, which might not be the case. So I think it actually makes sense to use switch in this case, because it is more flexible though cumbersome :).
Britt Green
2,611 PointsBritt Green
2,611 PointsMakes sense. Thanks! ;)