Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial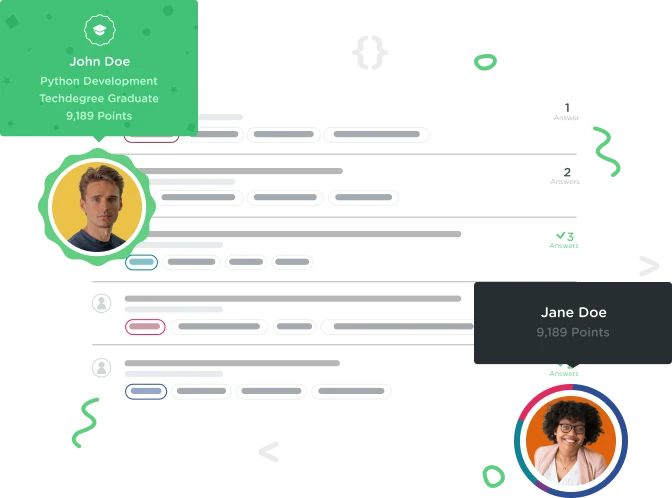

Carlos Pizarro
Courses Plus Student 1,878 PointsIs the logic of my function wrong? First challange of the IllegalArgumentExceptions
Is asking me to throw the the error exception if the amount of laps is minus to 0 but, does it mean that i should to equal the amount of bars to 0 and then throw the exception?
class GoKart {
public static final int MAX_BARS = 8;
private String color;
private int barCount;
private int lapsDriven;
public GoKart(String color) {
this.color = color;
}
public String getColor() {
return color;
}
public void charge() {
barCount = MAX_BARS;
}
public boolean isBatteryEmpty() {
return barCount == 0;
}
public boolean isFullyCharged() {
return MAX_BARS == barCount;
}
public void drive() {
if (barCount == 0) {
throw new IllegalArgumentException("Not enough battery");
}
}
public void drive(int laps) {
lapsDriven += laps;
barCount -= laps;
}
}
2 Answers
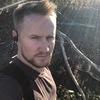
James Vlok
17,059 PointsYou need to check if there is enough battery power left to complete the amount of laps requested. So using an if statement in method drive(int laps). check if the number of laps is greater than the number of bars on the battery... eg..
if(barCount < laps){
// run this code if there is not enough battery power
//this is where you will throw your Exception in this case
throw new IllegalArgumentException("Not enough battery");
}
Now because you have a convince method drive() you will need to bubble up the Exception by declaring it as throwing.
public void drive() throws IllegalArgumentException{
drive(1);
}
below is a working code solution for the challenge hope this helps:
class GoKart {
public static final int MAX_BARS = 8;
private String color;
private int barCount;
private int lapsDriven;
public GoKart(String color) {
this.color = color;
}
public String getColor() {
return color;
}
public void charge() {
barCount = MAX_BARS;
}
public boolean isBatteryEmpty() {
return barCount == 0;
}
public boolean isFullyCharged() {
return MAX_BARS == barCount;
}
public void drive() throws IllegalArgumentException{
drive(1);
}
public void drive(int laps) throws IllegalArgumentException{
if(barCount < laps){
throw new IllegalArgumentException("Not enough battery");
}
lapsDriven += laps;
barCount -= laps;
}
}

Kevin Vaccianna
6,026 PointsCan you please Explain why the IllegalArgumentException is behind the drive(int laps)?
Carlos Pizarro
Courses Plus Student 1,878 PointsCarlos Pizarro
Courses Plus Student 1,878 PointsIt worked!! Awesome! Thank you so much!! :D PD: I need more practice