Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial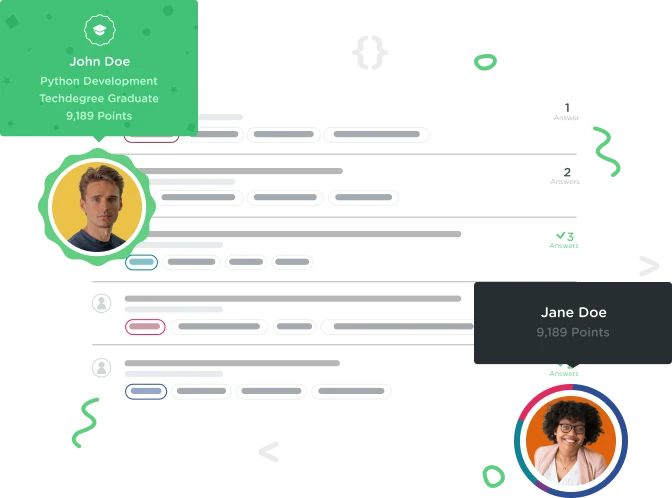
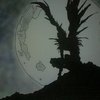
Lucas Santos
19,315 PointsIs the main purpose of "Casting" to allow you to share methods and properties between child and parent classes?
So I understand Up Casting and Down Casting and I see how it works changing the types of an object (type conversion). But now what is the main point of Casting in Java?
I believe I have a good idea but im not understanding 100% so I need to ask if the main idea behind casting is so that you can share Methods and Properties between parent and child classes?
1 Answer
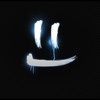
Grigorij Schleifer
10,365 PointsHi Lucas,
if you need to share methods and variables between parent and child classes you can use the principle of INHERITANCE. So if your subclass inherits from a superclass the methods and variables of the super class can be used by the subclass object too.
Like this:
// a superclass with a method
public class Bicycle {
public void drive() {
System.out.println("I am driving a fancy Bicycle");
}
}
..........................................
// a subclass that inherits from superclass
public class MountainBike extends Bicycle {
}
..........................................
// your Test class where the sublass calls the superclass method
public class Test {
public static void main(String[]arg) {
MountainBike mnt1 = new MountainBike();
// the drive() method from the super class can be used by subclass
mnt1.drive();
}
}
Typecasting can be used If you create a method that needs a Mountainbike as argument, but you give it an Object. You need do cast your Object to Mountainbike to by accepted by your method.
Like this:
public class MountainBike{
public void drive(MountainBike bike) {
System.out.println("I am driving a fancy Mountainbike");
}
}
...........................................
public class Test {
public static void main(String[]arg) {
Object obj = new Object();
MountainBike mnt1 = new MountainBike();
mnt1.drive((MountainBike) obj);
}
}
Here is a great link:
[ http://www.informit.com/articles/article.aspx?p=1932926&seqNum=5 ]
Let me know if my explanation is not valuable for you :)
Grigorij
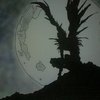
Lucas Santos
19,315 Pointsyeah I got the inheritance part down just the point of casting confused me because I don't see how it could have been used. Thanks
Grigorij Schleifer
10,365 PointsGrigorij Schleifer
10,365 PointsHey,
I hope I could help a little :)