Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial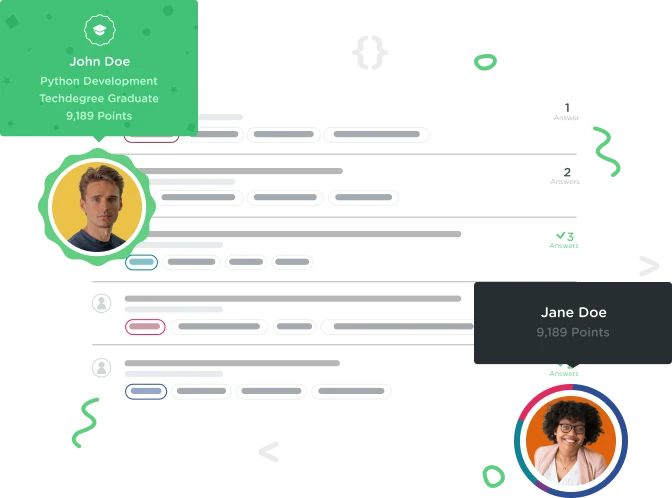
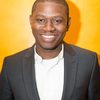
Welby Obeng
20,340 PointsIs there a better way to solve this Python Code Challenge?
Create a function named combo() that takes two iterables and returns a list of tuples. Each tuple should hold the first item in each list, then the second set, then the third, and so on. Assume the iterables will be the same length.
# combo(['swallow', 'snake', 'parrot'], 'abc')
# Output:
# [('swallow', 'a'), ('snake', 'b'), ('parrot', 'c')]
# If you use list.append(), you'll want to pass it a tuple of new values.
# Using enumerate() here can save you a variable or two.
def combo(arg1,arg2):
final = []
arg2_list=list(arg2)
for arg1_key,arg1_value in enumerate(arg1):
for arg2_key,arg2_value in enumerate(arg2_list):
if arg1_key == arg2_key:
final.append((arg1_value,arg2_value))
return final
3 Answers
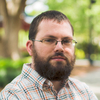
Kenneth Love
Treehouse Guest TeacherYou don't need to turn arg2
into a list. It's going to be an iterable so you can loop over it anyway.
I'm not sure why you're doing two for
loops. Using .enumerate()
is smart and gives you the index position. Use the value from one of the lists and use the index to get the same indexed value from the other list. Put those into a tuple in the right order and send 'em back.

Mike Tribe
4,114 Pointsdef combo(iter1, iter2):
output = []
for index, value in enumerate(iter1):
output.append((value, iter2[index]))
return(output)
William Li
Courses Plus Student 26,868 PointsHi, Welby, Here's my solution, it makes use of list comprehension and Python's built-in zip function.
def combo(iter1, iter2):
return [(i, j) for i, j in zip(iter1, iter2)]
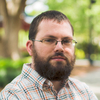
Kenneth Love
Treehouse Guest TeacherWhy go through so much trouble? zip()
does exactly what I want combo()
to do, so just do combo = zip
or
def combo(iter1, iter2):
return zip(iter1, iter2)
William Li
Courses Plus Student 26,868 PointsThanks, Kenneth, combo = zip
is great. btw, are you planning on doing a course on Advanced Python features? Functional programming, generator, iterator, decorator ... etc? I think such course will be an awesome addition to the current Python library at Treehouse.
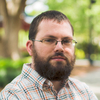
Kenneth Love
Treehouse Guest TeacherAlso, the workshop I did a few months ago on Python functional programming should be available already if you have a Pro membership or soon if you only have Basic.
Welby Obeng
20,340 PointsWelby Obeng
20,340 Pointscan you give me your answer explaining each line please?
Kenneth Love
Treehouse Guest TeacherKenneth Love
Treehouse Guest TeacherI'm not going to give you the entire answer but I'll lead you most of the way there.
You'll still need to return
output
but that should get you most of the way there.