Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial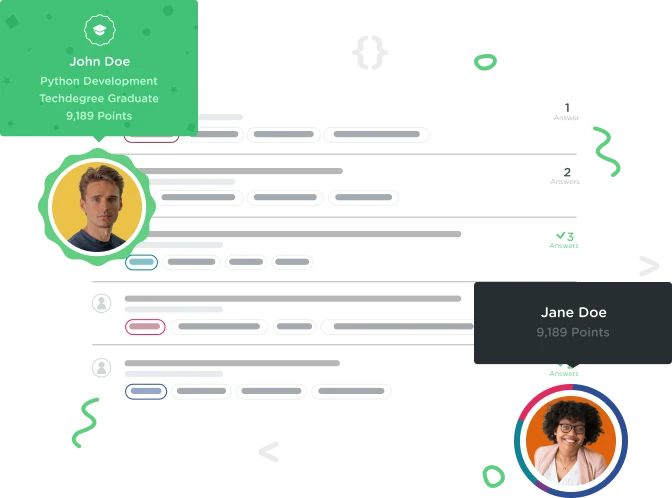
Raiyan Ahmad
3,622 PointsIs there a cleaner way to represent this?
I'm trying to always learn to write cleaner code, and I feel this code could be cleaner. Thanks
1 Answer

Jon Mirow
9,864 PointsHi there!
Do you mean task 1 or task 2?
In task 1, that's a pretty clean way to do it, although in real life you probably wouldn't be this specific unless you knew where you were adding in the item. For example, say you wanted to move the first item to the last index. There's a problem - because you pop the element off the list, the list shrinks before you call list.insert() on it. If you then tried to use the index of the last element in the original list to insert you'd get an Index error. Actually when you think about it, if you try and move the item in the list anywhere with a greater index, you'll have to reduce the index you give to insert. Personally in real life, without knowing the circumstances, I'd probably do something like:
def move(frm, to, lst):
"""
Moves an element in a list.
frm: index to move element from
to : index to move element to
lst: the list to be updated
"""
# As this function could be a spoiler to the challenge,
# It's code is at the pastebin linked below
pass
a_list = list(range(5))
move(0, 1, a_list)
print(a_list)
>>>[1, 0, 2, 3, 4]
function definition: https://pastebin.com/SgHkR7Tv !!Warning contains code that could solve the task!!
Sure it's a whole function, rather than a 1 liner, but to me it's cleaner when we're talking about general use.
As for task 2, we can have a bit more fun. What the question's essentially asking us, is to remove all elements from a list that don't match a certain rule. Python has a built in function just for this situation - filter().
To use filter, we need an iterable, and a function that can be applied to every element in the iterable and return true if we want to keep it, or false if we want to filter it out. In this example we want to remove everything that is not an integer, so we could to:
def is_int(element):
return isinstance(element, int)
And to use it, we could do something like:
a_list = ["1", "2", "3", 1, 2, 3, ["i"], {"ii": "iii"}]
b_list = list(filter(is_int, a_list))
Or of course, we could just assign it back to a_list if we didn't want to keep the original list.
Finally, if this was something we were only doing one time, and didn't need to make use of the re-usability of declaring a function, we can implement our is_int function as a lambda function when we call filter:
a_list = ["a", "b", "C", 1, 2, 3, ["i"], {"ii": "iii"}]
b_list = list(filter(lambda x: isinstance(x, int), a_list))
(I've posted these codes here are the task specifically asks you to use remove and del, so I don't think it counts as a spoiler)
Hope it helps! :)
Jason Anello
Courses Plus Student 94,610 PointsJason Anello
Courses Plus Student 94,610 PointsI don't see any code.