Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial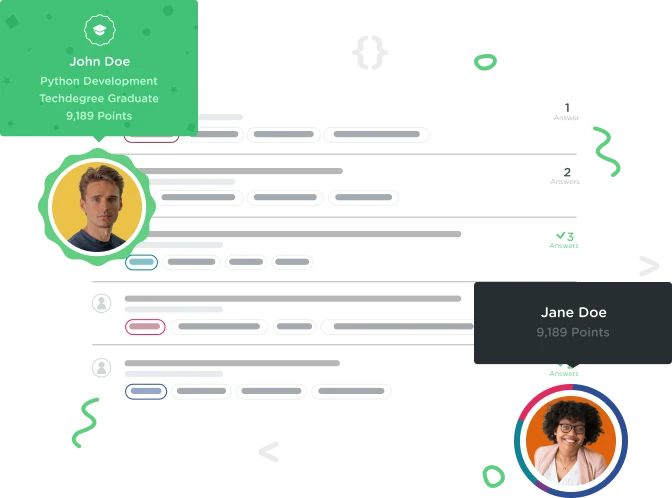
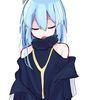
Kurobe Kuro^T_T^
5,369 Pointsis there a order with elif and else ???
please ....
3 Answers
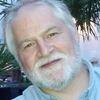
Jeff Muday
Treehouse Moderator 28,720 PointsYes... there is definitely an order to this because the blocks run sequentially. You can have one "IF" condition, many "ELIF" conditions, and one "ELSE". When you have "ELIF" blocks, it is important to note that the FIRST condition that is True will run, and the rest of the blocks are skipped.
Now... because of the indentation of the code blocks, Python supports infinitely nested IF/ELIF/ELSE sttructures.
The simplest case:
if condition1:
code block executes when condition1 is True
A typical case:
if condition1:
code block executes when condition1 is True
else:
code block executes when condition1 was False
A more complex case:
if condition1:
code block executes if condition1 is True
elif condition2:
code block executes if condition2 is True (note: if a previous condition was true, this block is skipped)
elif condition3:
code block executes if condition3 is True (note: if a previous condition was true, this block is skipped)
else:
code block executes if none of the above conditions were True
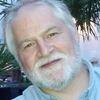
Jeff Muday
Treehouse Moderator 28,720 PointsThis example should explain it:
import random
# run the loop five times
for i in range(1,6):
# get a random age between 1 and 6
age = random.randint(1, 6)
# print it out so we can see what the random age was
print("-----")
print("test # {}".format(i))
print("Your actual age is {} years old".format(age))
# test the age if less than or equal to three
if age <= 3:
print("test result: Your age is less than or equal to three.")
else:
print("test result: You are older than three years.")
Now... we run the program to see it work.
-----
test # 1
Your actual age is 3 years old
test result: Your age is less than or equal to three.
-----
test # 2
Your actual age is 6 years old
test result: You are older than three years.
-----
test # 3
Your actual age is 2 years old
test result: Your age is less than or equal to three.
-----
test # 4
Your actual age is 5 years old
test result: You are older than three years.
-----
test # 5
Your actual age is 5 years old
test result: You are older than three years.
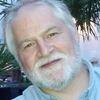
Jeff Muday
Treehouse Moderator 28,720 PointsBlue DiamondXD you are correct: it startes with one IF, ends with one ELSE (though this is optional), multiple ELIF conditions which are sandwiched in the middle.
This site might be helpful:
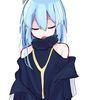
Kurobe Kuro^T_T^
5,369 Pointsthank you very much one more question when your putting ang age string like if age <= 3: print ("apptional{}".format(age)) dose it alway need to be after the alse or before the else
Kurobe Kuro^T_T^
5,369 PointsKurobe Kuro^T_T^
5,369 Pointsso is first if but only one if condition and elif is second that can have mutible condition andthen else is like if only one condition
but can if have multible lines of if and else and elif