Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial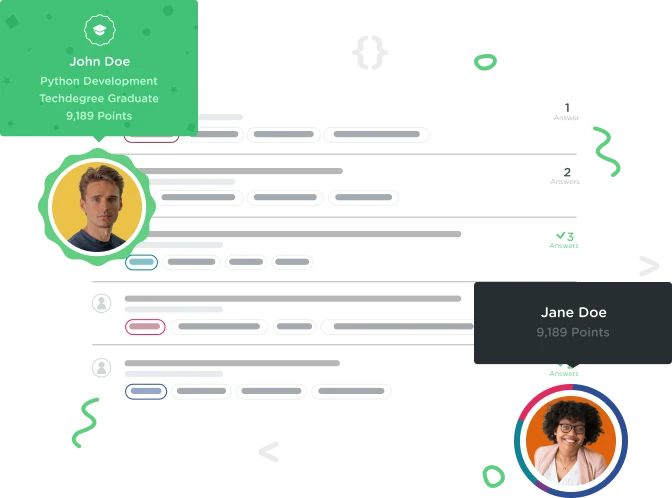

Chris Hummel
13,960 PointsIs there a reason we don't import the entire sqlalchemy base?
I noticed that Megan only imports part of sqlalchemy for the project. I was wondering why that is. Versus just doing import sqlalchemy and getting the entire repo should you need it.
2 Answers
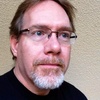
Chris Freeman
Treehouse Moderator 68,441 PointsHey Chris Hummel, a good question! There are a few factors being balanced: code readability, code writing speed, and possible some minor execution speed.
- code readability: If the only import was
import sqlalchemy
, then every reference into that library would require the code to list the full path to the Class, method, or attribute. Using more direct import references places the names into the current module namespace for direct access. Less cluttered code is perhaps more readable.
# Using a simple import
import sqlalchemy
Base = sqlalchemy.ext.declarative.declarative_base()
class Book(Base):
__tablename__ = "books"
id = sqlachemy.Column(sqlachemy.Integer, primary_key = True)
title = sqlalchemy.Column("Title", sqlachemy.String)
# Using more direct references allows direct access in module namespace
from sqlalchemy import (Column, Integer, String)
from sqlalchemy.ext.declarative import delclarative_base
Base = declarative_base()
class Book(Base):
__tablename__ = "books"
id = Column(Integer, primary_key = True)
title = Column("Title", String)
Some argue code that using direct references that are too specific makes it necessary to scan the imports to determine whether an object with a simple reference is local to the module or has been imported.
code writing speed. Certainly, only have to type a shorted reference to an imported object is better than having to type the full path to an import. Sometimes aliasing is used to short the names further:
import numpy as np
. Now onlynp
is needed.code execution speed. Each time an object is referenced, it needs to be looked up in the various namespaces, local to global, then builtins, to find the object.
Take Column
vs sqlalchemy.Column
for example. If imported as from sqlachemy import Column
then the look up would be:
* looking for "Column"
* look local to class Book
* look global in current module (Found "Column")
If imported as import sqlachemy
then the look up would be:
*:looking for "sqlalchemy.Column"
* look local to class Book
* look global in current module (Found "sqlalchemy")
* look in sqlalchemy (Found "Column")
The extra lookup into sqlalchemy
for Column
would happen every time the sqlalchemy.Column
is referenced. So a minor speed up would occur by eliminating the extra lookup. This speed up is almost insignificant in this case. In cases where the "dot" referenced name is used in a large loop then the extra lookup steps would be repeated a significant number of times.
Each project or group may have their own style of importing. The best rule is to follow along the current style. If developing your own modules then you get to establish the style.
Post back if you have more questions. Good luck!!

Chris Hummel
13,960 PointsSo would it be a good idea to lookup a library when working on a project to make sure you're only importing the pieces you are likely to use? Or would there be some cases where having the whole library would just be best. ie csv/json
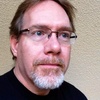
Chris Freeman
Treehouse Moderator 68,441 PointsItβs really about readability and in a minor way about speed. The βsizeβ of the import is not a significant factor as youβre only loading references (dict-like namespaces).
For json.loads
, itβs usually not used multiple times in the code so speed isnβt a factor (plus the I/O is Slooow). Using just loads
might be a misinterpreted name. Also using json.loads
is very common and might be confusing as to why the the module name was left off.
But all this is just guidelines, your project or team might set different or other specific guidelines.