Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial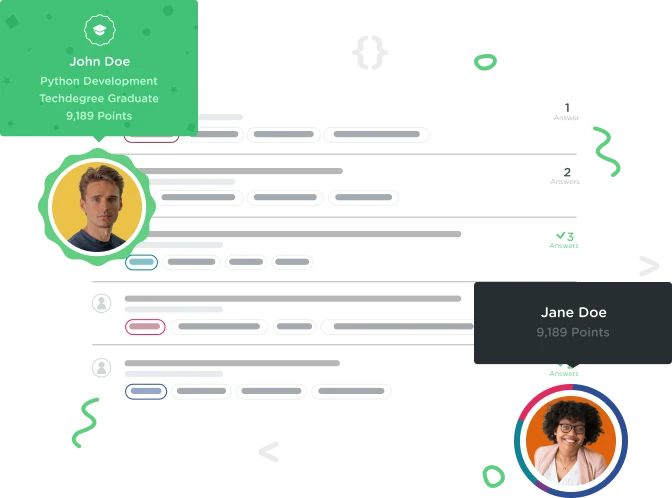

mohsinali4
2,988 PointsIs there a simple way to convert number words into integers?
Hi,
So I'm wondering if there is a simple way to convert number words (e.g. five, six, seventeen, etc.) into integers to be used by my Number Game app? I googled this and found most solutions going way over my head, so I was wondering if there is a simple way to do it based off the "Python Basics" covered thus far. Just wondering out of curiosity...my app works, but if someone enters in "five" the except ValueError kicks in (assuming "5" is not the random number generated) and says "five is NOT a number! Try again." So, it's not accurate to that degree. Here's what I have:
import random
def game():
secret_num = random.randint(1, 10)
guesses = []
while len(guesses) < 5:
user_input = input("Guess a number between 1 and 10: ")
try:
guess = int(user_input)
except ValueError:
print("{} is NOT a number! Try again.".format(user_input))
# compare guess to secret number
else:
if guess == secret_num:
print("Hit, my number was {}".format(secret_num))
break
elif guess < secret_num:
print("My number is higher than {}.".format(guess))
else:
print("My number is lower than {}.".format(guess))
guesses.append(guess)
else:
print("You didn't get it! My number was {}.".format(secret_num))
play_again = input("Do you want to play again? Y/N ")
if play_again.lower() == 'y':
game()
else:
print("Bye!")
game()
1 Answer
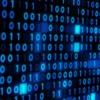
Alexander Davison
65,469 PointsIt's difficult to do so, since in English has many words and is a tricky language (although it is my main language I speak). It would take:
some_input = input("Enter a number: ").lower()
if some_input == "one":
some_input = 1
if some_input == "two":
some_input = 2
if some_input == "three":
some_input = 3
if some_input == "four":
some_input = 4
if some_input == "five":
some_input = 5
# and so on and so forth...
It would actually take that much work to do it since there's no way to convert English words into numbers out of thin air. There might be some resources out the to do this job, however it's really rare (at least I think) because nobody wants to do all that useless work.
So no, there's no simple way to do this job, and I don't recommend doing it.
Nice suggestion, though! :)
Good luck! ~alex