Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial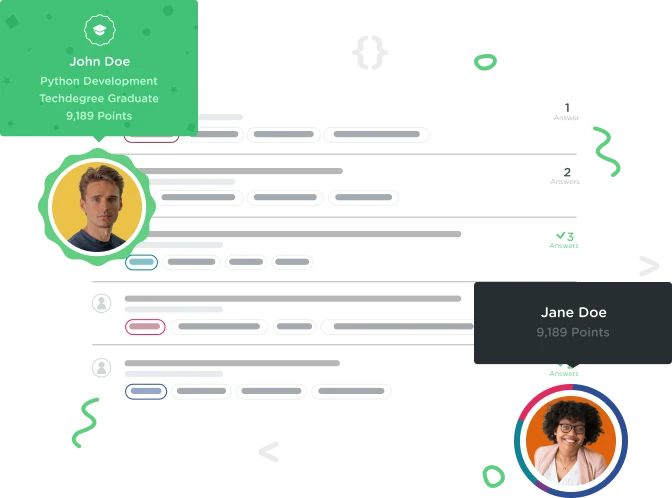

Tom Price
5,670 PointsIs there a simpler way of doing this (without having to replace strings)?
At the bottom of the code I have a variable called rank. I originally tried to update the string this way, pretty much the way you might with a number:
Eg:
var rank = ' '; if (score === 5) { Rank = ' gold crown';
It didn't work, and after a bit of Googling I ended up coming across the replace string method, which you can see below. The code now works but I was just wondering if there was a way of doing something similar without needing to resort to replace string. (That is also without simply using document.write () or alert () for each condition. Thanks in advance!
//starting score
var score = 0;
// question 1
var question1 = prompt('What is the capital of the UK?');
if (question1.toLowerCase() === 'london') {
score += 1;
}
// question 2
var question2 = prompt('What is the capital of Spain');
if (question2.toLowerCase() === 'madrid') {
score += 1;
}
// question 3
var question3 = prompt('What is the capital of France');
if (question3.toLowerCase() === 'paris') {
score += 1;
}
// question 4
var question4 = prompt('What is the capital of Germany');
if (question4.toLowerCase() === 'berlin') {
score += 1;
}
// question 5
var question5 = prompt('What is the capital of Italy');
if (question5.toLowerCase() === 'rome') {
score += 1;
}
//rank
var rank = 'default';
if (score === 5) {
var newRank = rank.replace('default', ' gold crown');
} else if (score <5 && score > 2) {
var newRank = rank.replace('default', ' silver crown');
} else if (score > 0 && score < 3) {
var newRank = rank.replace('default', ' bronze crown');
} else {
var newRank = rank.replace('default', '...actually, you scored too lowly to receive a rank. Sorry!');
}
var sentence = '<h3>You got a score of ' + score + ' out of 5. You achieved the rank of' + newRank + '.</h3>.'
document.write (sentence);
console.log ('End program');
4 Answers
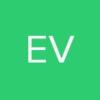
Evans Owino
24,888 PointsHi Tom,
Your original method of updating the string should have worked.
Can you confirm whether or not you used "rank" instead of "Rank" as per your example (as they would be two different variables)?
'var rank = ' '; if (score === 5) { Rank = ' gold crown';
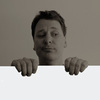
Sean T. Unwin
28,690 PointsHi Tom Price
In your second post, each of the rank = ...
statements within the conditionals have a right bracket before the line-ending semi-colon. If you remove those brackets that particular block of code should work and could, therefore, be substituted for the rank.replace()
statements in your original post.
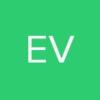
Evans Owino
24,888 PointsHi Tom,
I am sure it was just a minor syntactic error that prevented your code from working.
To check, you can test it in your browser of choice by typing "about:blank" in the URL bar and typing your code in the console and running it (usually helps me check my sanity :) ).
Hope this helps.

Tom Price
5,670 PointsAhh that bracket is just there because I copy and pasted the code from my first post and forgot to delete them from the end of the line. The original code didn't include them, but still didn't work.
Anyway, it all works fine now, so I have absolutely no idea what I was doing wrong the first time round. Really appreciate you both helping out, by the way. There are some super helpful people on here!
Tom Price
5,670 PointsTom Price
5,670 PointsThanks for taking a look, Evans. I'm pretty sure I wrote it out like this, originally:
The rank variable would always show up in the sentence as the original string, though. Not sure if it's because of some error I was making, or whether it's because it just doesn't work like that.