Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial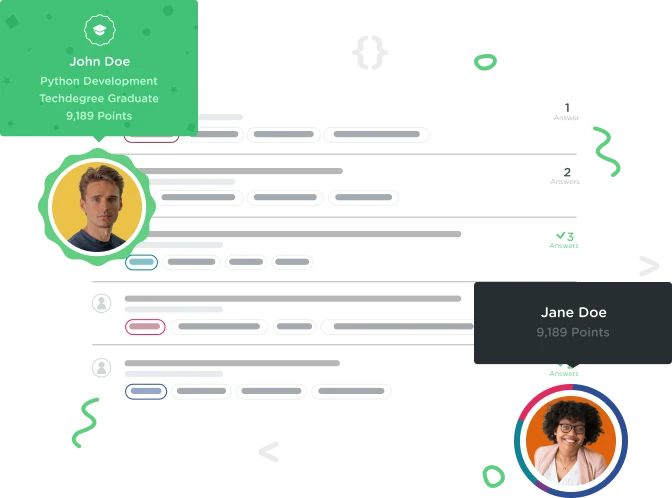

Nathalie Agnekil
6,665 PointsIs there a way to access the property name to be written out?
I did something a little different from what Dave did, and I get a list and all that, but I'd want to get the property name printed as well, not just the value of the property. How do I access this in an array of objects? My code is chaos so I can't print it but this is the jist of what I wanna do:
for (var property in students) { print("<h1>" + property + ": " + students[property].name + "</h1>");
You can see I want the final result to be for example "name: Hannah", but I can't get the property name "name" to write out. Whatever I do I get either "[object Object]: Hannah" or "0: Hannah". Or nothing at all.
1 Answer

James Anwyl
Full Stack JavaScript Techdegree Graduate 49,960 PointsHi Nathalie,
You can use the Object.keys() method to get the property names.
For example:
var allKeys = Object.keys(students[i]);
message += '<h1>' + allKeys + ':' + '</h1>';
This will get all the keys. To get a specific key you can use:
var nameKey = Object.keys(students[i])[0];
var trackKey = Object.keys(students[i])[1];
message += '<h1>' + nameKey + ':' + '</h1>';
message += '<h1>' + trackKey + ':' + '</h1>';
Full student_report.js code:
var message = '';
var student;
function print(message) {
var outputDiv = document.getElementById('output');
outputDiv.innerHTML = message;
}
for (var i = 0; i < students.length; i += 1) {
student = students[i]
message += '<h2>Student: ' + student.name + '</h2>';
message += '<p>Track: ' + student.track + '</p>';
message += '<p>Points ' + student.points + '</p>';
message += '<p>Achievements: ' + student.achievements + '</p>';
var allKeys = Object.keys(students[i]);
var nameKey = Object.keys(students[i])[0];
var trackKey = Object.keys(students[i])[1];
message += '<h1>' + allKeys + ':' + '</h1>';
message += '<h1>' + nameKey + ':' + '</h1>';
message += '<h1>' + trackKey + ':' + '</h1>';
}
print(message);
Hope this helps :)