Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial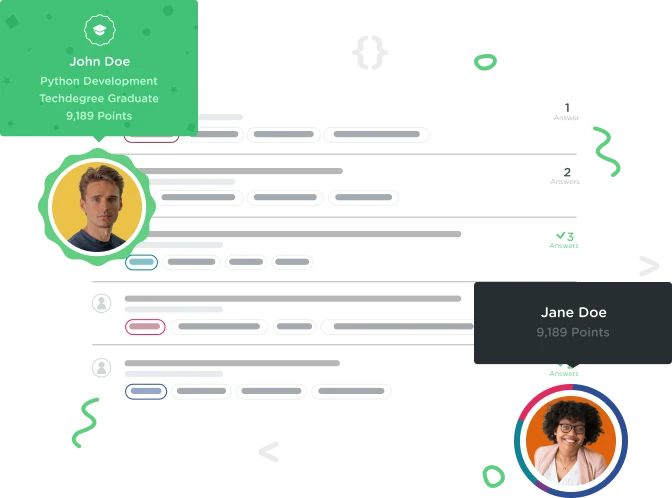

larsberg
58,676 PointsIs there a way to append multiple items to an array using .append in Swift?
Just curious if there's a way to append multiple items to an array using .append in Swift on the same line instead of separate lines.
5 Answers

Stone Preston
42,016 Pointsyou can append an array to the end of another array:
Using +=
var cityArray: String[] = ["Portland","San Francisco","Cupertino","Seattle"]
cityArray += ["Vancouver", "Los Angeles", "Eugene"]
Using .append
var cityArray: String[] = ["Portland","San Francisco","Cupertino","Seattle"]
cityArray.append(["Vancouver", "Los Angeles", "Eugene"])
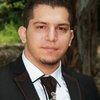
David Anton
Courses Plus Student 30,936 PointsHey Lars,
if you declare an array named -> var items = ["item1","item2"] you can add multi items like this:
items += ["item3","item4","item5"]
the result should be : ["item1","item2","item3","item4","item5"]

Srinivasan Senthil
2,266 Pointsvar appendexample1 : [String] = ["Air", "Water", "Fire", "Earth"] // This will be your answer for the above line: ["Air", "Water", "Fire", "Earth"] // lets append an array element called "Spirit" to the above array so called "appendexample1"
appendexample1.append("Spirit") // This will be the example for the above statement. ["Air", "Water", "Fire", "Earth", "Spirit"]

Srinivasan Senthil
2,266 PointsMaybe this will help. Just thought of another direction.
var arrayexample3 : [Int] = [1,2,3,4,5] var arrayexample4 : [Int] = [6,7,8,9,10] arrayexample4 = arrayexample3 + arrayexample4 arrayexample4 += arrayexample3
Try it out. But i dont think append will work for entire set of array contents. Still not sure.

Srinivasan Senthil
2,266 Pointsyou cant add more elements using append method. iOS Swift Book You can add a new item to the end of an array by calling the array’s append method:
shoppingList.append("Flour") // shoppingList now contains 3 items, and someone is making pancakes Alternatively, append an array of one or more compatible items with the addition assignment operator (+=):
shoppingList += ["Baking Powder"] // shoppingList now contains 4 items shoppingList += ["Chocolate Spread", "Cheese", "Butter"] // shoppingList now contains 7 items
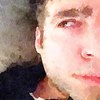
Osiris Maldonado
699 PointsTwo things:
1) Thanks for your great examples. They quite clear and helpful. 2) Awesome coffee mug!
Reading this was a very nice refresher to the array section.
Osiris Maldonado
699 PointsOsiris Maldonado
699 PointsDid something in the .append syntax change recently? Your .append solution doesn't seem to work. I am also interested in knowing how to add an array of elements using .append.
Thanks!
Srinivasan Senthil
2,266 PointsSrinivasan Senthil
2,266 PointsHi Osiris Maldonado,
Small syntax error in the example above. var cityArray: [String] = ["Portland","San Francisco","Cupertino","Seattle"] // Replaced the brackets properly above.
The below is not possible. cityArray.append(["Vancouver", "Los Angeles", "Eugene"])