Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial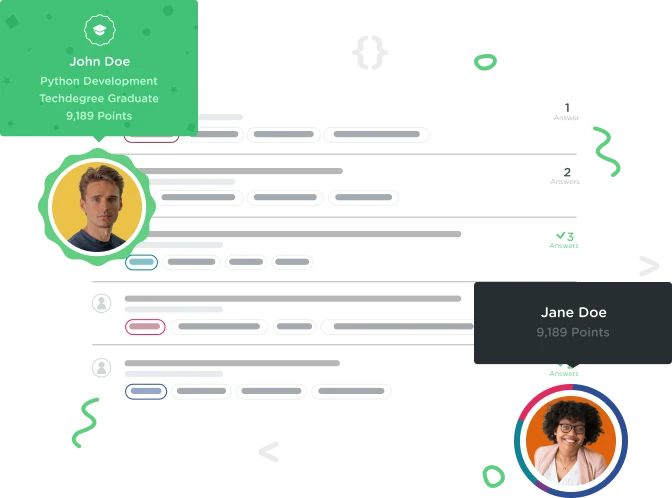

akshaan Mazumdar
997 PointsIs there a way to check the data types in a loop ?
I can use del and remove the string and list and boolean one by one. But is there a way i could run the list through a loop and check the items for their data type????
messy_list = ["a", 2, 3, 1, False, [1, 2, 3]]
# Your code goes below here
X=messy_list.pop(3)
messy_list.insert(0,X)
2 Answers
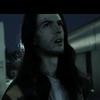
codeoverload
24,260 PointsThis is how I would do it:
messy_list = ["a", 2, 3, 1, False, [1, 2, 3]]
# Your code goes below here
messy_list.insert(0, messy_list.pop(messy_list.index(1)))
copy_list = messy_list[:] # Make a copy of messy_list
for item in copy_list: # Iterate over copy_list
if type(item) is not int:
del messy_list[messy_list.index(item)] # if the item isn't an int remove it from messy_list
You need to make a copy of messy_list because you can't remove items from a list while iterating over it.
If you've still got questions, don't hesitate to ask me :)
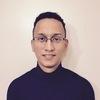
Daniel Santos
34,969 PointsThis is a functional way of solving this problem. It doesn't require explicit copy of the list and its just one line. Of course, don't give up readability for less code. I just wanted to show another way of doing it and if it makes sense for you, even better.
messy_list = ["a", 2, 3, 1, False, [1, 2, 3]]
# filter will iterate through every element in messy_list, and you can access them with x.
# If type(x) is int evaluates to True, then whatever element x is a the moment will be included
# in the new list.
clean_list = list(filter(lambda x: type(x) is int, messy_list))
print(clean_list)
This is a more functional programming style, if you have any questions, please let me know.
-Dan
Daniel Santos
34,969 PointsDaniel Santos
34,969 PointsI wouldn't make a copy. If the list is huge, then your program will not perform well. Here is what I would do:
Check my official answer, which is another way of doing this.
codeoverload
24,260 Pointscodeoverload
24,260 PointsFrom a performance perspective you're certainly right :)
I just wanted to modify the messy_list directly because of the code challenge.
But still thanks for your suggestion!