Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial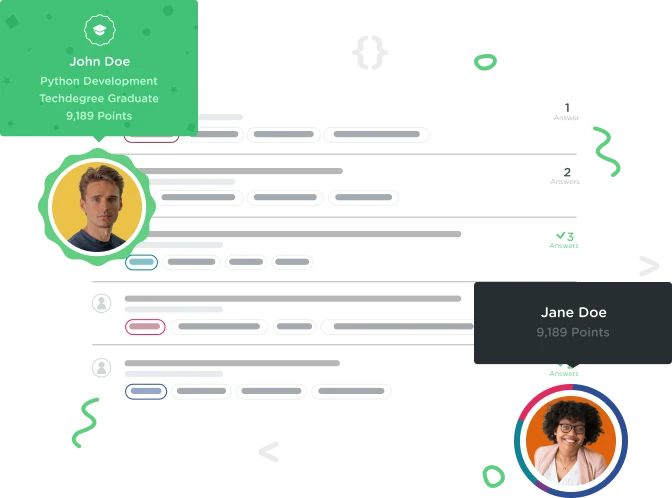
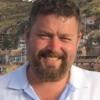
Michael Dowd
16,272 PointsIs there a way to repopulate the fields with JS?
So, in step one of this phase we were given an additional challenge to hide certain titles and genres from the select box when a particular category was selected. I did this and was pretty proud of myself. But in this section we just use php to repopulate the select boxes, I thought there would be an easier way with JS by recording the values in a JS variable then putting them back in when the page reloads, but I (finally) realized that as the page reloads so does my JS and my variables are back to zero (or undefined or something). So my questions are: 1) Is this what AJAX is all about? 2) Is there some way/trick to pass a PHP variable to a JS variable.
3 Answers
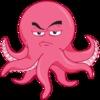
Kieran Black
9,139 PointsHi Michael,
Glad you have been bitten by the programming bug, you should indeed be proud of your efforts thus far.
As you discovered when your page reloads your javascript will in essence reset itself, that is, it will return to the state it was in on first load. It will not persist the information between loads and it is your job to program the page to be able to load correctly with the correct information upon a refresh, a form submission that hits an error etc.
AJAX is a way of fetching data from the server while the page has been loaded already, perhaps to get a list of town names for a town drop down when the user changes the county, perhaps to get the latest tweets from david beckham and render them on screen. It can fetch this data in the background and return the data to your javascript for processing.
There are no tricks in passing a PHP variable to a JS one, just different strategies people may use. Here are some:
Use an AJAX request to query the server and return a JSON object with the information you need, you can then use this information within the flow of your JS program.
Create a JS variable within the code of your page and have PHP put its value within this variable, when the page loads it will have your value.
Build the elements of your page using HTML and replicate the DOM within the HTML you output, for instance if you have an item selected in a dropdown list then within the PHP also make this select option selected. If you have a checkbox ticked then code it so it is ticked again. Then when the javascript runs it can take off from the current state.
There is no hard and fast rule for how things are done, it is up to you to try and design something that is robust. This comes with a lot of trial and error.
Hope this helps a bit
KB

matthewharrington2
Courses Plus Student 13,219 PointsYou can use JS to store values in local storage(browser memory/Web Storage) if you want them to persist through page loads. Something like this, before reload, would save the input field value(id="name") and store it to a browser memory/Web Storage variable.
var formName = document.getElementById("name").value;
localStorage.setItem("userName",formName);
After a page reload you could use this to reset the name field:
document.getElementById("name").value = localStorage.getItem("userName");
Local storage shouldn't be used to store any sensitive info though such as passwords etc(https://www.owasp.org/index.php/HTML5_Security_Cheat_Sheet#Local_Storage) but it can be used for situations like these where you want info to persist. Also look into sessionStorage which persists only as long as a window or tab is open as opposed to local storage that has to be deleted with something like localStorage.clear(); This can be a very useful tool in HTML5 web apps and other user interactive platforms.
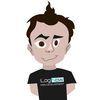
Ilia Loginov
13,015 PointsThere's no need to use local storage. Here's my solution:
<tr>
<th><label for="format">Format</label></th>
<td>
<select id="format" name="format" disabled>
<option value="">Select One</option>
<?php
if (isset($format)) {
echo '<option value="' . $format . '" selected>' . $format . '</option>';
}
?>
</select>
</td>
</tr>
<tr>
<th>
<label for="genre">Genre</label>
</th>
<td>
<select name="genre" id="genre" disabled>
<option value="">Select One</option>
<?php
if (isset($genre)) {
echo '<option value="' . $genre . '" selected>' . $genre . '</option>';
}
?>
</select>
</td>
</tr>
const format = {
Books: [
'Audio',
'Ebook',
'Hardback',
'Paperback',
],
Movies: [
'Blu-ray',
'DVD',
'Streaming',
'VHS',
],
Music: [
'Cassette',
'CD',
'MP3',
'Vinyl',
]
};
const genre = {
Books: [
'Action',
'Adventure',
'Comedy',
'Fantasy',
'Historical',
'Historical Fiction',
'Horror',
'Magical Realism',
'Mystery',
'Paranoid',
'Philosophical',
'Political',
'Romance',
'Saga',
'Satire',
'Sci-Fi',
'Tech',
'Thriller',
'Urban',
],
Movies: [
'Action',
'Adventure',
'Animation',
'Biography',
'Comedy',
'Crime',
'Documentary',
'Drama',
'Family',
'Fantasy',
'Film-Noir',
'History',
'Horror',
'Musical',
'Mystery',
'Romance',
'Sci-Fi',
'Sport',
'Thriller',
'War',
'Western',
],
Music: [
'Alternative',
'Blues',
'Classical',
'Country',
'Dance',
'Easy Listening',
'Electronic',
'Folk',
'Hip Hop/Rap',
'Inspirational/Gospel',
'Jazz',
'Latin',
'New Age',
'Opera',
'Pop',
'R&B/Soul',
'Reggae',
'Rock',
]
};
const categorySelector = $('#category');
const formatSelector = $('#format');
const genreSelector = $('#genre');
function changeOptions(selector, category, selected = null) {
if (category) {
if (selector.attr('disabled')) selector.removeAttr('disabled');
selector.html('<option value="">Select One</option>');
eval(selector.attr('name'))[category].forEach(value => {
let select = '';
if (selected) {
if (selected === value) select = 'selected';
}
selector.append(`<option value="${value}" ${select}>${value}</option>`);
});
} else {
selector.html('<option value="">Select One</option>');
selector.attr('disabled', true);
}
}
$(document).ready(function() {
if (categorySelector.val()) {
const category = categorySelector.val();
const selectedFormat = formatSelector.val();
const selectedGenre = genreSelector.val();
if (selectedFormat) changeOptions(formatSelector, category, selectedFormat);
if (selectedGenre) changeOptions(genreSelector, category, selectedGenre);
}
});
categorySelector.change( (event) => {
const category = $(event.target).val();
changeOptions(formatSelector, category);
changeOptions(genreSelector, category);
});