Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial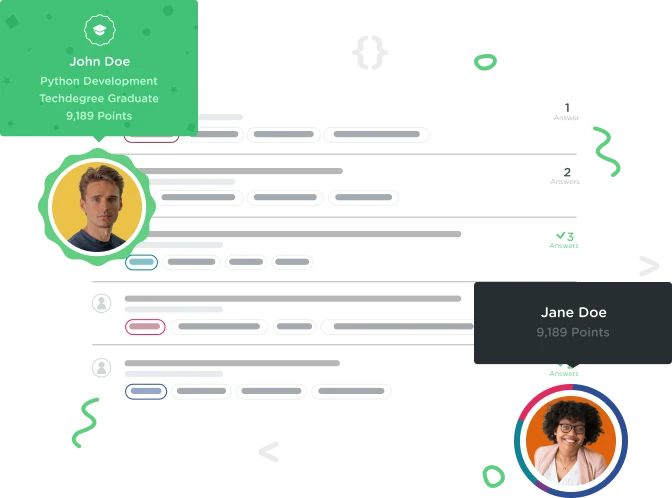
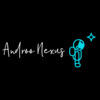
androonexus
Courses Plus Student 2,528 PointsIs there a way to set a new property to all objects in an array of objects without looping?
I've been practicing with arrays in Javascript, and I created an issue for myself to try to solve. I have an array of objects that I created:
const fruit = [
{
name : 'Apple',
price : 1.50,
quantity : 17,
},
{
name : 'Banana',
price : 2.50,
quantity : 21,
},
{
name : 'Pear',
price : 4.50,
quantity : 11,
},
{
name : 'Strawberry',
price : 5.50,
quantity : 7,
},
];
I would like to have a total inventory value for all available stock. I need to add a new property to each object that multiplies quantity and price. Even though my array only has 4 elements, I tried to imagine what I would do if it was 1000 elements. So far in what I have learned loops are best for this.
for (let i = 0; i < fruit.length; i++) {
fruit[i].inventoryValue = fruit[i].price * fruit[i].quantity;
}
let totalInventoryValue = 0;
for (let i = 0; i < fruit.length; i++) {
totalInventoryValue += fruit[i].inventoryValue;
}
console.log(`The total inventory value for all items is ${totalInventoryValue}`);
This code gave me the answer to my made up issue for practice. But my question is for real world programming. Is it efficient to have a loop set a property to all objects in an array of objects? I'm thinking about how companies that have thousands of users add a feature to user accounts, for example. Every time they add a feature to a user object, do they create and update a loop with new features that weren't there initially? Or, is there some way to set a property to all objects without looping. What is the most efficient way to accomplish what my code does? Thanks for your time.
1 Answer
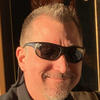
Peter Vann
36,427 PointsHi androonexus!
I'm going to do my best to answer this conceptually and not with a code example, and hopefully, it will suffice.
If not, I can also try to provide an example, too, eventually.
I think you'd want to instantiate your individual "user" objects from a class.
Then just make inventoryValue a new class attribute (or method).
That way, all you'd have to do is update the class and all instances will automatically be updated.
Does that make sense?
Actually, I did come up with a simple example.
Consider this code:
class Car {
constructor(name, year) {
this.name = name;
this.year = year;
}
}
car1 = new Car("Ford", 2014);
car2 = new Car("Fiat", 2009);
console.log(car1.name + " " + car1.year);
console.log(car2.name + " " + car2.year);
Later, you could update it to add a new id attribute, like this:
class Car {
constructor(name, year) {
this.name = name;
this.year = year;
this.id = this.name + " " + this.year; // New attribute
}
}
car1 = new Car("Ford", 2014);
car2 = new Car("Fiat", 2009);
console.log(car1.id);
console.log(car2.id);
Both cases would log:
Ford 2014
Fiat 2009
And then, to build your array of objects, do something like this instead:
const cars = [];
car1 = new Car("Ford", 2014);
car2 = new Car("Fiat", 2009);
car3 = new Car("VW", 1987);
cars.push(car1);
cars.push(car2);
cars.push(car3);
for (let car of cars) {
console.log("Car ID: " + car.id);
}
Etc...
Which will log out:
Car ID: Ford 2014
Car ID: Fiat 2009
Car ID: VW 1987
It's similar to using constants and other variables in your code.
For example, you declare this at the beginning of your code:
const TICKET_PRICE = 35;
Then you reference TICKET_PRICE a 1000 places in your code, such as:
const charge = quantity * TICKET_PRICE;
If you change the TICKET_PRICE to 40, it will automatically update it everywhere (all 1000 places) it is referenced.
I hope that helps.
Stay safe and happy coding!