Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial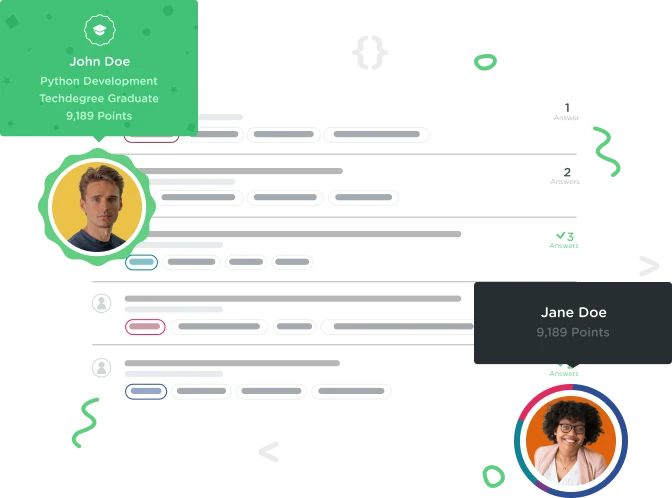

Rodrigo Mac Lean
Courses Plus Student 402 PointsIs there a way to use a variable in a single string value accompanied with text?
So I tried to use my variable the following way: print("Hello, label_tag", but it only printed the label tag, and not what I defined it to be. The only way I managed to used the defined label was writing the following: print("Hello,", label_tag), is that the only way a variable can be put into a string (as a separate value)?
2 Answers
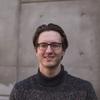
Kai Kovacik
5,769 PointsThere are two other methods that I would recommend. The first of which is concatenation which is accomplished via the '+' character. Here is how it would look in your code:
print("Hello, " + label_tag),
you can concatenate as many strings together as you'd like in one line! Here's an example:
my_number = 4
print("My number is: " + str(my_number) + ". What's yours?")
Your output would be:
My number is: 4. What's yours?
Another method which is less pretty but much more efficient is to use the .'format'. Here is an example:
my_number = 4
print("My number is: {}. What's yours?".format(my_number))
This will output the same thing as before. The way that format works is it replaces the '{}' in your string with the variable that you input. Note that the inputted variable does not have to be a string as format will convert it for you. if you want to add multiple format elements you can simply add more '{}' into your string:
my_number = 4
your_number = 7
print("My number is: {}. Your number is {}.".format(my_number, your_number))
This will output:
My number is: 4. Your number is 7.
As you can see it will just replace the '{}' elements from left to right. There is also a lot more functionality that I'm not going to get into with this method, but you can feel free to check it out here: https://docs.python.org/3.4/library/string.html

Rodrigo Mac Lean
Courses Plus Student 402 PointsThank you Kai! I think I'll use the .format way!
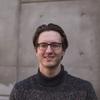
Kai Kovacik
5,769 PointsYou're welcome Rodrigo! Happy Coding!
Grigorij Schleifer
10,365 PointsGrigorij Schleifer
10,365 PointsThis is a great answer! Thank you also for me ...