Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial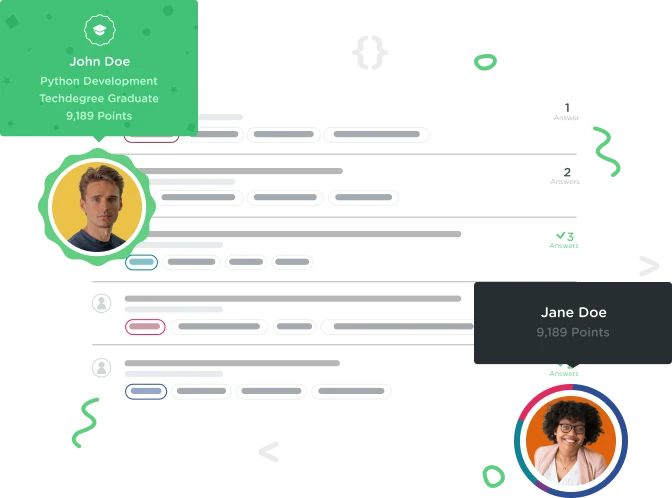
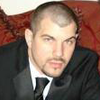
Gary Calhoun
10,317 PointsIs there a way to write an If Null statement?
I noticed if I don't enter a number and just press enter it still picks the random number. Is there a way to have a failsafe where a user is required to enter number or
if (Null){
alert("Hey! You didn't enter a number!");
}
I know that anything that is inputted is considered a string before its converting so an empty string how do we deal with it? Hope I make sense.
4 Answers
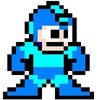
Robert Richey
Courses Plus Student 16,352 PointsHi Gary,
When getting input from the user, if they just press enter without typing in anything, the return value is an empty string. You can check for that inside a loop until the user types something before pressing enter.
var input = "";
while (input === "") {
input = prompt("Type something");
}
// once outside the loop, input contains something other than an empty string
Cheers
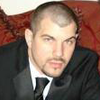
Gary Calhoun
10,317 PointsOh ok a while loop like php. Thanks for the example.
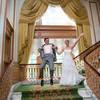
Colin Bell
29,679 PointsIf you wanted a number specifically, and only a number, you could do something like this:
do{
var ask = parseInt(prompt("Please enter a number"), 10);
}while(isNaN(ask));
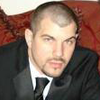
Gary Calhoun
10,317 PointsIs the 10 in your example a substitute for a persons number they guessed?
do{
var userNumber = [0, 1, 2, 3, 4, 5, 6];
var ask = parseInt(prompt("Please enter a number"), userNumber);
}while(isNaN(ask));
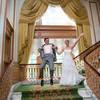
Colin Bell
29,679 PointsThe 10
is the radix
parameter of parseInt()
. It means it will parse it to a base 10 number (the standard numeral system).
If you only wanted binary numbers - 1s or 0s - you would use 2.
MDN suggests to always add in the radix parameter:
Always specify this parameter to eliminate reader confusion and to guarantee predictable behavior. Different implementations produce different results when a radix is not specified.
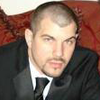
Gary Calhoun
10,317 PointsThanks ok so if I understand correctly the 10 is standard 0-9 in numerics and the hexadecimal is numbers and letters which we don't want since we only want a number to be submitted, octal is 0-7 even though is deprecated.
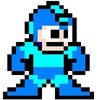
Robert Richey
Courses Plus Student 16,352 PointsAlways specify this parameter to eliminate reader confusion and to guarantee predictable behavior. Different implementations produce different results when a radix is not specified.
What this means is that browsers can, and do, implement ECMA Script (aka JavaScript) differently. Google's Chrome uses the V8 JavaScript engine; Firefox uses SpiderMonkey; and so on.
These engines are not exactly the same, so different browsers can produce different results from the same JavaScript code.
Robert Richey
Courses Plus Student 16,352 PointsRobert Richey
Courses Plus Student 16,352 PointsTo directly answer your question: Is there a way to write an If Null statement?
You can compare a variable to the keyword
null
.It's worth noting that if you declare a variable, without giving it a value, it's value is
undefined
.