Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial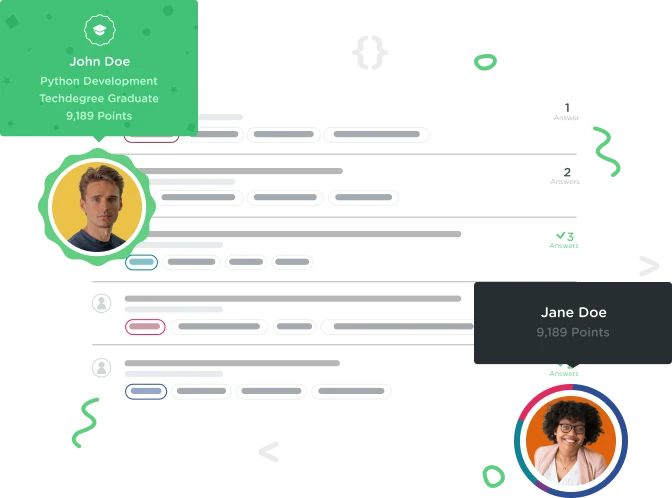
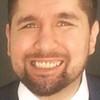
William Daugherty
Full Stack JavaScript Techdegree Graduate 30,935 PointsIs there an issue with the locale detection for Argentina("es-AR")?
This same issue stumped me in the previous challenge when I had to code the conversion function for just Argentina but now the code that was coded in for the challenge itself is having the same issue.
java.lang.AssertionError: Expected: is "First is $1.00 (USD) which is $15,48 (Argentine Peso)" but: was "First is $1.00 (USD) which is $ 15,48 (Argentine Peso)"
here's the code
'''java package com.teamtreehouse.challenges.homes;
import com.teamtreehouse.challenges.homes.model.HousingRecord; import com.teamtreehouse.challenges.homes.service.HousingRecordService;
import java.math.BigDecimal; import java.text.NumberFormat; import java.util.Comparator; import java.util.Currency; import java.util.List; import java.util.Locale; import java.util.Optional; import java.util.OptionalInt; import java.util.function.Function; import java.util.stream.Collectors; import java.util.stream.IntStream;
public class Main { public static void main(String[] args) { HousingRecordService service = new HousingRecordService(); List<HousingRecord> records = service.getAllHousingRecords();
// The first housing record is a summary of all of the United States
HousingRecord usRecord = records.get(0);
System.out.print("Hardcoded price converter method: ");
Function<Integer, String> argentinaPriceConverter = getArgentinaPriceConverter();
String priceConversion = getPriceConversionForRecord(
usRecord,
argentinaPriceConverter);
System.out.println(priceConversion);
System.out.print("Higher Order Function price converter");
String closurePriceConversion = getPriceConversionForRecord(
usRecord,
createPriceConverter(Locale.forLanguageTag("es-AR"), new BigDecimal("15.48"))
);
System.out.println(closurePriceConversion);
// Once you get the `createPriceConverter` method working, it'll work for any country!
System.out.println("Higher Order Function showing off:");
getConvertedPriceStatements(records, createPriceConverter(
Locale.CANADA,
new BigDecimal("1.35")
)).forEach(System.out::println);
}
public static Function<Integer, String> getArgentinaPriceConverter() { Locale argLocale = Locale.forLanguageTag("es-AR"); // This fluctuates and is hardcoded temporarily BigDecimal argPesoToUsdRate = new BigDecimal("15.48");
Function<Integer, BigDecimal> usdToArgentinePesoConverter =
usd -> argPesoToUsdRate.multiply(new BigDecimal(usd));
Function<BigDecimal, String> argentineCurrencyFormatter = price -> {
Currency currentCurrency = Currency.getInstance(argLocale);
NumberFormat currencyFormatter =
NumberFormat.getCurrencyInstance(argLocale);
return String.format("%s (%s)",
currencyFormatter.format(price),
currentCurrency.getDisplayName()
);
};
return usdToArgentinePesoConverter.andThen(argentineCurrencyFormatter);
}
public static Function<Integer, String> createPriceConverter(Locale locale, BigDecimal usdRate) {
// TODO: Examine the hardcoded getArgentinaPriceConverter
method
// TODO: Using the arguments passed into this method return a function that will work for any locale and rate
return usdPrice -> {
BigDecimal foreignRate = usdRate.multiply(BigDecimal.valueOf(usdPrice));
NumberFormat converter = NumberFormat.getCurrencyInstance(locale);
Currency displayConverter = Currency.getInstance(locale);
return String.format("%s (%s)",
converter.format(foreignRate),
displayConverter.getDisplayName()
);
};
}
public static String getPriceConversionForRecord(HousingRecord record, Function<Integer, String> priceConverter) { NumberFormat usFormatter = NumberFormat.getCurrencyInstance(Locale.US); return String.format("%s is %s (USD) which is %s", record.getRegionName(), usFormatter.format(record.getCurrentHomeValueIndex()), priceConverter.apply(record.getCurrentHomeValueIndex()) ); } '''
2 Answers

Steven Branson
Courses Plus Student 4,211 PointsI also had this issue. I worked around it with by replacing the currencyFormatter
in the argentineCurrencyFormatter
function with this static method:
static String fakeFormatter(BigDecimal price) {
String pString = String.valueOf(price);
return String.format("$%s,%s",
pString.substring(0, pString.indexOf('.')),
pString.substring(pString.indexOf('.') + 1, pString.indexOf('.') + 3));
}
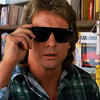
Nathaniel Meyer
8,175 PointsNo, the issue isn't with the locale detection. The issue is with Treehouse's hamcrest test. Don't break perfectly good code to make it pass broken validation.
Nathaniel Meyer
8,175 PointsNathaniel Meyer
8,175 PointsSometimes tests are broken. You shouldn't break your code to make it pass broken validation.
The Java library returns Argentine currency in the format $ 999.999.999,99 and the assignment says, "These functions are in working order". Nothing about the assignment says we're supposed to override the Java library.
Their validation is borked and they should fix it.