Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial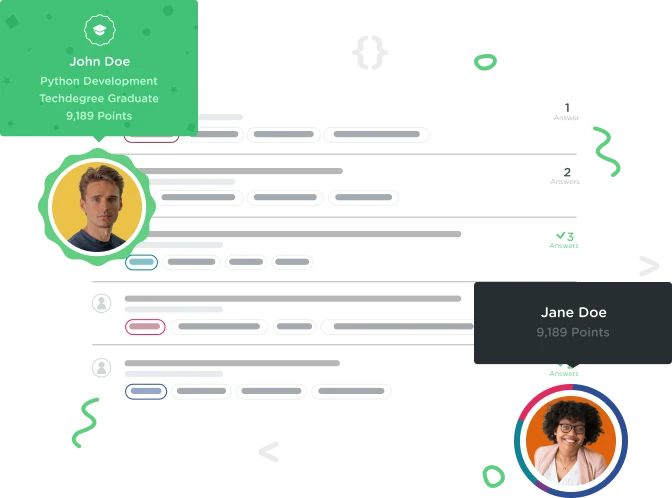

emhes
6,934 PointsIs there another challenge I can try to practice this concept?
I've been moving along through JavaScript basics without any issues until this challenge.
I understand how to write a function with two parameters and how to call that function. I even played around with a bit of my own code on one of the earlier stages to make sure I had a good understanding of parameters and returns:
function getAverageFeet(num1, num2, num3) { var average = (num1 + num2 + num3) / 3; return average; }
console.log(parseInt(getAverageFeet(5, 50, 150)) + "-feet");
...but this challenge completely threw me. I think because I wasn't comfortable with where to put the 'upper' & 'lower' parameters within the random number math formula.
I'd really like to make sure I'm understanding everything up to this point, is there another similar challenge I can take on that doesn't include the random number formula?
Thanks.
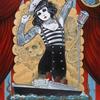
Saira Bottemuller
Courses Plus Student 1,749 Pointsthis is a good question, Emilie, I was hoping for the same thing. Dave did a great job with the teaching, it's just going to take me some getting used to with that formula. in my case, i think it would help if i could see a a breakdown of what each piece of the formula is and WHY it goes where it does. at least for me anyway. thank you for your response John!
1 Answer
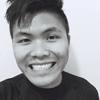
John Domingo
9,058 PointsLet's take a look at the following formula:
Math.floor(Math.random() * (6 - 1 + 1)) + 1;
If we break it down one by one, we see that the outermost operation is Math.floor(x) + 1
. The floor function works by rounding down a float regardless of the decimal places. For example 1.0000001 rounds down to 1 and 1.9999999 also rounds down to 1. Floor() basically truncates everything to the right of the decimal place if you want to think of it that way.
Then, what ever x is, it is simply rounded down and then 1 is added to the truncated number. I will explain why the 1 is added after I explain the inner funciton (i.e., Math.random() * (6 - 1 + 1)
)
Math.random() generates a random number between 0 and 1, including 0 but not including 1. So it can go from 0 up to 0.9999999999...
If we simply apply the floor function to just the generated random number, the result will always be 0 because Math.random()
only generates numbers greater than or equal to 0 and less than 1. This is why we multiply random numbers with larger numbers to scale the result up so it's not always zero.
Let's look at ten numbers generated by Math.random() * 10
:
0: 0.00757 * 10 = 0.0757
1: 0.70006 * 10 = 7.0006
2: 0.14752 * 10 = 1.4752
3: 0.90371 * 10 = 9.0371
4: 0.23131 * 10 = 2.3131
5: 0.78659 * 10 = 7.8659
6: 0.23670 * 10 = 2.3670
7: 0.81528 * 10 = 8.1528
8: 0.51122 * 10 = 5.1122
9: 0.43452 * 10 = 4.3452
Notice how each value generated by the random function will round down to zero but when multiplied by 10, the numbers vary between 0 and 9 (see below):
0: Math.floor(0.0757) = 0
1: Math.floor(7.0006) = 7
2: Math.floor(1.4752) = 1
3: Math.floor(9.0371) = 9
4: Math.floor(2.3131) = 2
5: Math.floor(7.8659) = 7
6: Math.floor(2.3670) = 2
7: Math.floor(8.1528) = 8
8: Math.floor(5.1122) = 5
9: Math.floor(4.3452) = 4
So now we see that by multplying by 10 we can get a random number between 0 and 10 (including 0 but not 10). Now if we add 1 to whatever is generated we will always have a number between 1 and 10 (both 1 and 10 included)!!
You can see here that 10 sets the upper limit on the random function (remember the excercise?) so basically what ever you multiply with Math.random()
becomes its upper limit. Adding 1 to the product is what makes the upper limit inclusive or exclusive.
Now the tricky part is understanding the latter part of Math.floor(Math.random() * (6 - 1 + 1)) + 1
.
Think of it as Math.floor(Math.random() * (upper - lower + 1)) + lower
;
Say we want a value between 6 and 10. The lower limit is 6 and upper limit is going to be 10. The random function will be multiplied by 5 (see below):
upper - lower + 1 : (10 - 6) + 1 = 5
This means that the left term will always return 0, 1, 2, 3, or 4. Remember 5 will not be included since Math.random() only returns 0 to 0.99999999999...
Now add the lower limit to 0, 1, 2, 3, or 4:
lower = 6
0 + lower = 6
1 + lower = 7
2 + lower = 8
3 + lower = 9
4 + lower = 10
So the whole formula will always give 6, 7, 8, 9, or 10! It's just a little math trick that's complicating the formula but hopefully it makes sense now.
When it comes to trying to parse out what a formula does or how it works, the best thing I've learned (as I mentioned in my previous post!!) is to handwrite your code and manually calculate your expected results just like I did here. This will flush out all the values you should expect and eventually, you should see a number pattern that solves your problem.
Happy coding!

Iain Simmons
Treehouse Moderator 32,305 PointsJohn Domingo, it seems a lot of people are struggling with this formula. Thanks so much for breaking it down, using examples, and explaining it very well!
John Domingo
9,058 PointsJohn Domingo
9,058 PointsHi Emilie, I am not sure what concept you want more practice on. Perhaps you could help clarify.
If you want to learn more about functions, you might want to check out the Functions stage in the Javascript Foudnations course to learn the concept from a different teacher and try out different exercises.
This specific challenge is called the 'random number challenge' so I think there is little getting around the random number concept. It's a very powerful tool to understand and my best recommendation would be to handwrite your code and manually calculate your expected results. Recall exactly what Math.random() and Math.floor() do. Then try multiplying that results of the random function by values you would expect to come from the lower and upper parameters. Remember that these upper and lower values are supposed to bound the random number that is generated so try out simple numbers like 0 and 1 and see if your inspection helps you figure out what's going on.
There are many other great resources out there too and a quick little Google search will certainly lead you in the right direction.