Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial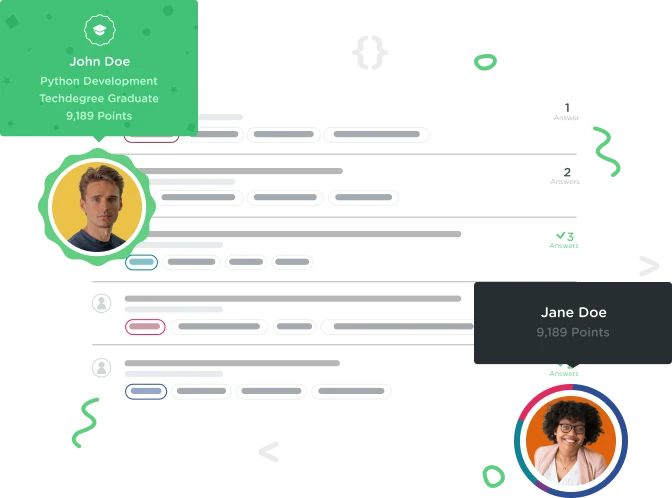
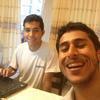
Wilfredo Casas
6,174 PointsIs there any advantage in using kwargs over other methods?
I did both examples of the video and I find the example 1 much easier and faster than the kwargs method, am I missing something?
Nala and Anubis are my dogs by the way
#Example 1:
class Nala:
def __init__(self, hit_points = 1, color = "brown", weapon = "bite", cry = "guau"):
self.hit_points = hit_points
self.color = color
self.weapon = weapon
self.cry = cry
#Example 2:
class Anubis:
def __init__(self, **kwargs):
self.hit_points = kwargs.get("hit_points", 3)
self.color = kwargs.get("color", "black")
self.weapon = kwargs.get("weapon", "paw")
self.cry = kwargs.get("cry", "arrr")
2 Answers
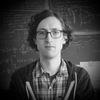
Joshua Yoerger
11,206 PointsI am still very much a neophyte when it comes to correct practice and programming "pythonically," so I'll just summarize and include some links at the end that I found helpful. I imagine one of the more experienced pythonistas here will be able to add more as well.
From what I can tell, *args and **kwargs should be used only when there is a really good reason to do so, because defining attributes explicitly lends itself to better OOP. However, variable-length argument lists can be very useful when using generic decorators or wrappers since the outside function needn't know about the arguments being passed to the inner function. Another good reason I see mentioned online frequently is when you have a function that connects with a database and you really do need to pass different arguments depending on the situation or type of DB being connected to - something that might come up when working with flask and peewee for example.
I found this excerpt from Effective Python very informative and the top answer on this SO question was particularly helpful to me. Hope that helps!
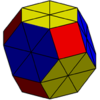
Jeff Hartl
Python Web Development Techdegree Student 8,420 PointsThanks Chris Freeman for recommending Example 1. It is more explicit, and easier to read and understand.
In the video, Kenneth's justification for example 2 seemed to be that it was somehow "simpler", with less code, but both examples seemed to me to involve nearly the same amount of keystrokes from the person writing the code.
Thanks everyone for the further explanations.
Martin Cornejo Saavedra
18,132 PointsMartin Cornejo Saavedra
18,132 PointsThat was very clear to me, thanks.
Chris Freeman
Treehouse Moderator 68,458 PointsChris Freeman
Treehouse Moderator 68,458 PointsNicely put! Explicit is better than implicit -- Zen of Python (try
import this
)Wilfredo Casas
6,174 PointsWilfredo Casas
6,174 PointsThanks but I really didn't understand, that theory is too advanced for me still. What I wanted to know was if there was any benefit in using Example 2 over Example 1, since both have the same result.
Chris Freeman
Treehouse Moderator 68,458 PointsChris Freeman
Treehouse Moderator 68,458 PointsFor non advanced uses (and users) stick with Example 1.
One example of a potential pit fall when using
**kwargs
is not catching typos. Say you use Example2 but call it with:nub1 = Anubis(colour='pink')
nub1.color
would still be black.Wilfredo Casas
6,174 PointsWilfredo Casas
6,174 PointsAll right