Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial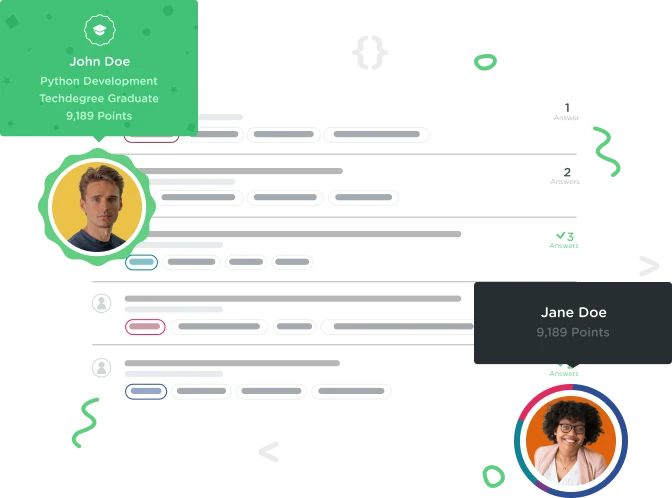
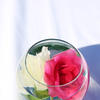
Carlos Marin
2,675 PointsIS there any other method I can use instead of QuerySelectorAll in this challenge?
I keep doing this challenge over and over again but it seems the only solution I was able to find was using the document.querySelectorAll method. I am trying to get to used to using the other methods of selecting in the DOM, but it seems like to select multiple elements with the other methods require a for loop. and when I try to use a for loop in this challenge it seems not to work. (I understand the challenge wants thenavigationLinks variable to store all the links.)
let navigationLinks = document.querySelectorAll('nav a');
let galleryLinks;
let footerImages;
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title>Nick Pettit | Designer</title>
<link rel="stylesheet" href="css/normalize.css">
<link href='http://fonts.googleapis.com/css?family=Changa+One|Open+Sans:400italic,700italic,400,700,800' rel='stylesheet' type='text/css'>
<link rel="stylesheet" href="css/main.css">
<link rel="stylesheet" href="css/responsive.css">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
</head>
<body>
<header>
<a href="index.html" id="logo">
<h1>Nick Pettit</h1>
<h2>Designer</h2>
</a>
<nav>
<ul>
<li><a href="index.html" class="selected">Portfolio</a></li>
<li><a href="about.html">About</a></li>
<li><a href="contact.html">Contact</a></li>
</ul>
</nav>
</header>
<div id="wrapper">
<section>
<ul id="gallery">
<li>
<a href="img/numbers-01.jpg">
<img src="img/numbers-01.jpg" alt="">
<p>Experimentation with color and texture.</p>
</a>
</li>
<li>
<a href="img/numbers-02.jpg">
<img src="img/numbers-02.jpg" alt="">
<p>Playing with blending modes in Photoshop.</p>
</a>
</li>
</ul>
</section>
<footer>
<a href="http://twitter.com/nickrp"><img src="img/twitter-wrap.png" alt="Twitter Logo" class="social-icon"></a>
<a href="http://facebook.com/nickpettit"><img src="img/facebook-wrap.png" alt="Facebook Logo" class="social-icon"></a>
<p>© 2016 Nick Pettit.</p>
</footer>
</div>
<script src="js/app.js"></script>
</body>
</html>
3 Answers

KRIS NIKOLAISEN
54,971 PointsAn alternate solution to querySelectorAll:
let navigationLinks = document.getElementsByTagName("nav")[0].getElementsByTagName("a");
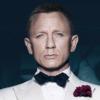
Aurelian Spodarec
10,801 PointsHow would you do it with class selector? Since it's a list of nodes, it won't work like querySelectorAll. So how would you extract that?
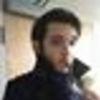
luther wardle
Full Stack JavaScript Techdegree Student 18,029 PointsI'm curious why you would want to use another selector? Even though querySelectorAll can be loose, in this case its very direct since you have <a> tags inside your <li> tags
A solution to make the code "tighter" if you were doing this for a project would be to simply divide your <nav> tags into <navOne><navTwo> or make a <div> around them to access them by interacting with the div instead of the nav tag itself. although understandably, that makes the variable's name misleading.
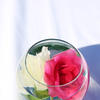
Carlos Marin
2,675 PointsI read that using the QuerySelector methods can be 'loose' and it is preferred to use a more direct approach. I was unable to complete the DOM selection practice, so I came back to reinforce my understanding of the DOM.
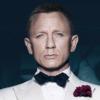
Aurelian Spodarec
10,801 PointsIt's simple...
Efficiency.
QuerySelectorAll looks for for 'ALL' items, while e.g. query selector class, looks for ONLY class items.
Guil talks about this, and it's best to use 'not' query selector all for efficiency purposes. It's easier to work with... but on a big project that can take it's hit.
Which means.. and the point of the question is... how do you do it with another selector?
It's the same story with re-inventing the wheel. Why would you do it? To learn.
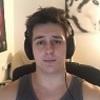
ale8k
8,299 PointsYou could grab them all under a new class/name
document.getElementsByClassName('[CLASS]');
// or name?
document.getElementsByName('[NAME]');
However depending on your program these can cause issues as they return a live HTML collection and querySelectors do not.
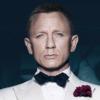
Aurelian Spodarec
10,801 PointsYou don't put brackets inside the selector though.
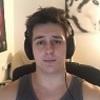
ale8k
8,299 PointsThe brackets are just representing what he could place there lol.
Aurelian Spodarec
10,801 PointsAurelian Spodarec
10,801 PointsQuery selector and e.g. 'getElementsByClassName' store/get the data differently.
So you would need to do more work. Look inside the DOM how the data is stored.