Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial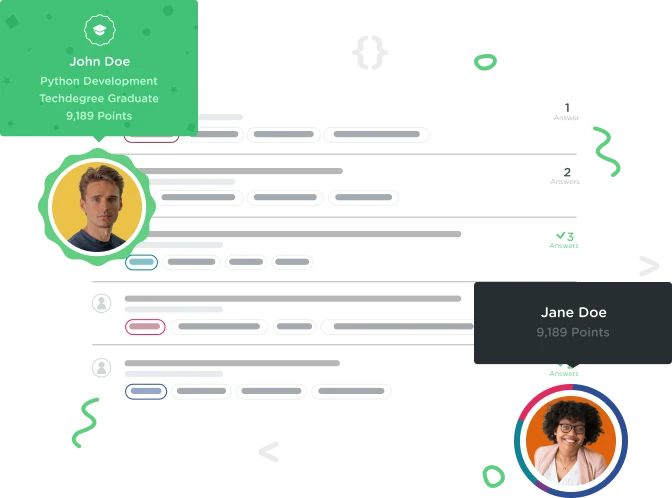

fahad lashari
7,693 PointsIs there any way around using so many if statements and while loops to run a programme? there has to be, right?
I am just wondering. There has to be a more efficient way of running functions on demand one at a time depending on the requestion of the user. To me it seems like a waste of memory that the function loops through every single 'if' statement until one is true and then does it again and again until it comes accross a 'break'.
Isn't there any way of excecuting just the part of the code that's needed dynamically. I am not an expert but a novice. However I have a feeling that there a more efficient ways of doing this.
If someone could shed a light on it i'd really appreciate it!
Thanks
2 Answers

fahad lashari
7,693 PointsHi guys. Thanks for the awesome guidance.
so I did some research and used what I've learnt so far in python and came up with this solution. It works perfectly but only excecutes functions if or when needed. It also uses only one loop which is to print the list and only 2 'if' statements. I believe this could be a better approach to solving this problem as using a large chain of 'if else' statement doesn't seem to efficient or optimal in my opinion (I could be totally wrong). Please try this in your workspaces and let me know if you encounter any errors. I haven't yet implemented any functionality to stop the user from typing the incorrect Values e.g. if you type in a string where the programme asks you to type the index of the item it will throw an error so just press enter or type a number but I will be adding . Here is my code:
import sys
shopping_list = []
command = input("""Would you like to start your supreme AI shopping experience?\n Y/n""")
def show_help():
print('\nSeperate each item with a comma.')
print('\nType DONE to quit, SHOW to see the current list, and HELP to get this message')
add_item()
def show_list():
count = 1
for item in shopping_list:
print('{}: {}'.format(count, item))
count+=1
add_item()
def add_item():
command = input('\nPlease enter the name of the item:\n')
if command != "DONE".lower() and command != "SHOW".lower() and command != "HELP".lower():
index = input("Add this to certain spot on the list?, "
"\ncurrently we are on number {}\nPRESS ENTER for last spot and continue or give me a NUMBER. NO LETTERS or " "SYMBOLS:\n".format(len(shopping_list)))
if index:
shopping_list.insert((int(index)-1), command.strip())
else:
shopping_list.append(command.strip())
add_item()
else:
function_dict[command]()
def exit():
print("""Thank for shopping with us.
I know it's a bit late but we dont really sell anything...\nThe shopping list looks neat though!\nOk bye now""")
sys.exit()
function_dict = {"Y".lower():add_item, "HELP".lower():show_help, "SHOW".lower():show_list, "DONE".lower():exit}
function_dict[command]()
I'd appreciate any feedback
kind regards,
Fahad
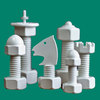
Steven Parker
231,275 Points
There are ways to optimize your code.
Alex is partly right, operations in a computer may be a lot more efficient than they seem in the code. But there are advanced techniques that can be used to replace long "if...else" chains when it matters. These may involve arrays, dictionaries, exceptions, comprehensions, and mapping functions. If you continue your Python learning here, you will see examples of some of these in more advanced courses. And others may be discovered with a little web browsing.
Happy coding!
Alex Arzamendi
10,042 PointsAlex Arzamendi
10,042 PointsNormally, functions are expected to be run during an event that triggers them such as being called or by something that a user does. So in the event of having something that is displayed when the user clicks a button or types something in a while statement or something like that you are pretty much left with no other option. It is actually quite efficient when compared to how(say) a web framework handles the method calling, they are always in memory waiting to be called which would mean that the resources are always allocated for you. Stacks of functions being called can be modified by certain programming paradigms, but when it comes to simple stuff there is simply no need to do so. One thing that you can do is implement something similar to a switch statement(I know that Python does not have one built in, but you can mimic the functionality, extra points if you find it and implement it! It is quite fun)