Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial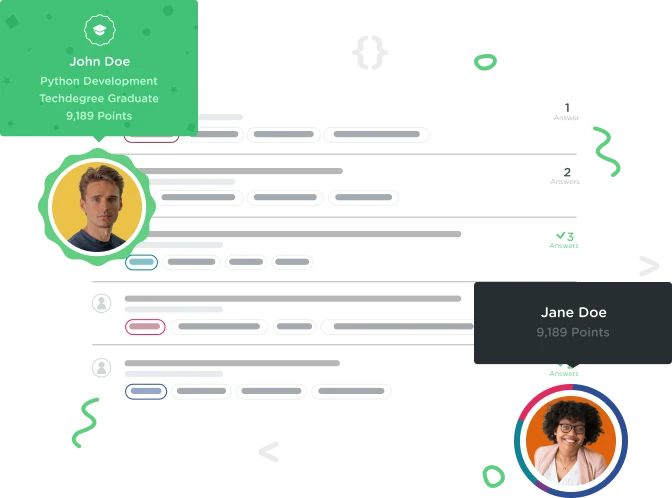

Nathalie Agnekil
6,665 PointsIs there any way to solve this with a for in-loop?
Well. I did the first part of the challenge with a for in-loop. Worked perfectly fine, but then when I was supposed to add the search-thing it got all messed up and nothing worked. First, my loop didn't even want to run more than once, then [i] was not defined (obviously since I didn't have a for-loop declaring my i), and then it just got messy.
Now, I know this code is messed up and you don't need to point out everything that's wrong, I just started adding EVERYTHING that Dave did but with the for in-loop instead, haha, and it obviously didn't work. But how can I do this with a for in-loop? (I'm just so very, very curious and refuse to give up on this even though it's said to use a for-loop for arrays :P There's gotta be a way!)
var search;
var student;
var message = '';
var students = [
{name: "Hannah", track: "Web Design", achievements: 45, points: 1034},
{name: "Darryll", track: "PHP", achievements: 56, points: 510},
{name: "Amy", track: "Ruby", achievements: 83, points: 5620},
{name: "Sandra", track: "C# and Java", achievements: 34, points: 9630},
{name: "Dylan", track: "Front End Developer", achievements: 23, points: 3068}
];
function print(message) {
document.write(message)
}
function studentList(student) {
var report = "<h1>Name: " + students[property].name + "</h1>";
report += "<p>Track: " + students[property].track + "</p>";
report += "<p>Achievements: " + students[property].achievements + "</p>";
report += "<p>Points: " + students[property].points + "</p>";
return report;
}
while (true) {
search = prompt("Who are you looking for? To exit, type 'quit'.");
if (search === null || search.toLowerCase() === "quit"){
break;
}
for (var property in students) {
student = students;
if(student.name === search.toLowerCase()) {
message = studentList(student);
print = message;
}
else {
print("We can't find who you're looking for.");
}
}
}
ยดยดยด
1 Answer

Jordan Hoover
Python Web Development Techdegree Graduate 59,268 PointsIf you're asking about the for loop specifically, you're not using the iterable. The first part of the for defines what you are calling each iteration (in my case 'student'). It should look like this:
for (var student in students) {
if (student.name === search.toLowerCase()) {
message = studentList(student);
print(message);
}