Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial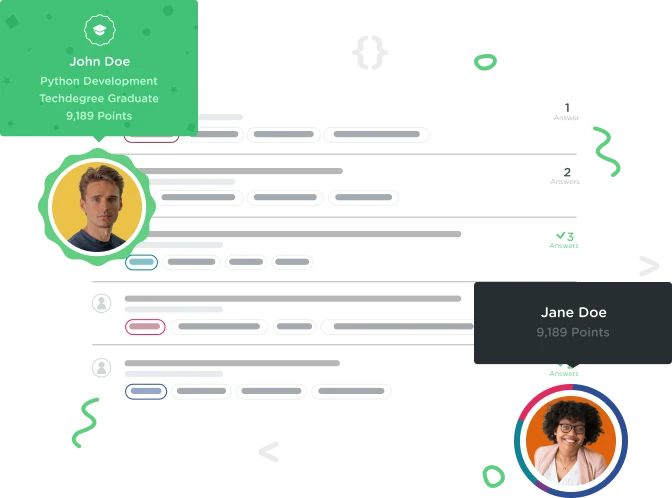

Michael Hampshire
12,206 PointsIs there anyway to refactor my code?
I'm working on a sample ATM project and I'm setting the button clicks to set a label to the button that was clicked. I have it working and everything is fine, but I'm thinking about DRY(Don't Repeat Yourself). So with that said, is there anyway I can refactor my code? This is done in Visual Studio 2015:
using System;
using System.Windows.Forms;
namespace atm
{
public partial class Form1 : Form
{
public Form1()
{
InitializeComponent();
lblAccountNumber.Text = "";
}
string pinNumber = "1212";
int[] buttonNumbers = { 1, 2, 3, 4, 5, 6, 7, 8, 9, 0};
private void btnNumber1_Click(object sender, EventArgs e)
{
lblAccountNumber.Text += buttonNumbers[0].ToString();
}
private void btnNumber2_Click(object sender, EventArgs e)
{
lblAccountNumber.Text += buttonNumbers[1].ToString();
}
private void btnNumber3_Click(object sender, EventArgs e)
{
lblAccountNumber.Text += buttonNumbers[2].ToString();
}
private void btnNumber4_Click(object sender, EventArgs e)
{
lblAccountNumber.Text += buttonNumbers[3].ToString();
}
private void btnNumber5_Click(object sender, EventArgs e)
{
lblAccountNumber.Text += buttonNumbers[4].ToString();
}
private void btnNumber6_Click(object sender, EventArgs e)
{
lblAccountNumber.Text += buttonNumbers[5].ToString();
}
private void btnNumber7_Click(object sender, EventArgs e)
{
lblAccountNumber.Text += buttonNumbers[6].ToString();
}
private void btnNumber8_Click(object sender, EventArgs e)
{
lblAccountNumber.Text += buttonNumbers[7].ToString();
}
private void btnNumber9_Click(object sender, EventArgs e)
{
lblAccountNumber.Text += buttonNumbers[8].ToString();
}
private void btnNumber0_Click(object sender, EventArgs e)
{
lblAccountNumber.Text += buttonNumbers[9].ToString();
}
}
}
2 Answers
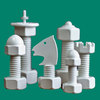
Steven Parker
231,110 Points
It looks like you could reduce this code significantly.
It appears that you could have all buttons share the same handler just by identifying the button within the handler and using the identity to select the string to append to the label text.
For example, if the button Id's all end in the character you wish to append:
private void button_Click(object sender, EventArgs e)
{
var btn = ((Button)sender).ID;
lblAccountNumber.Text += btn[btn.Length - 1];
}

Michael Hampshire
12,206 PointsNevermind I figured it out! Thank you!!
Michael Hampshire
12,206 PointsMichael Hampshire
12,206 PointsI was wondering if I could create a method that would replace all of the button_click methods instead of having 10 different methods for each button. If that makes sense. Sorry I'm still trying to learn C#.
P.S. Your method worked as well.
Michael Hampshire
12,206 PointsMichael Hampshire
12,206 PointsIf you don't mind, can you explain what is going on with the code inside the method?
Steven Parker
231,110 PointsSteven Parker
231,110 PointsSure, the first line casts the sender object to a Button (we know that a button must have caused the event) and then takes the "ID" property of the button assigning it to the string "btn". I'm expecting the ID will be something like "Button4".
Then, we take the last character of that string (using one less than the length as an index) and append that to the label's text. By using the ID of the button that was clicked (the "sender") to get this string, all buttons can share the same handler.
Happy coding!