Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial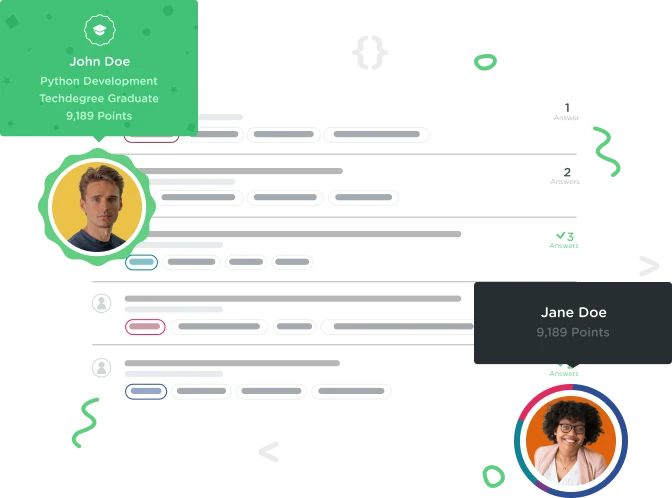

fmquaglia
11,060 PointsIs there some sort of performance drawback if you decide to keep using the self keyword?
Is there some sort of performance drawback (or any other problem or code smell) if you decide to keep using the self keyword to refer to the instance's properties within your methods even if there isn't any naming conflict?
E.G.:
class Product {
let title : String
let price : Double
init(title: String, price: Double) {
self.title = title
self.price = price
}
func discountedPrice(percentage: Double) -> Double {
return price - price * percentage / 100
}
}
vs.
class Product {
let title : String
let price : Double
init(title: String, price: Double) {
self.title = title
self.price = price
}
func discountedPrice(percentage: Double) -> Double {
return self.price - self.price * percentage / 100
}
}
2 Answers
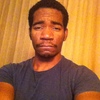
Stephen Whitfield
16,771 PointsThere shouldn't be a drawback... especially if it's a derivative from objective-C for accessing class properties and also Apple's preferred method for accessing class properties.

X. Szuh
2,486 PointsSelf refers to an instance of an object. I think you are misunderstanding why it is needed.

fmquaglia
11,060 PointsHi Deanne Chance ,
No, not at all. I come from a Ruby background, where the keyword to refer to the object itself is also "self". So it's pretty clear to me what self is about. "Know your self" ;-)
Even further I use to create a variable in OO Javascript called to self, to refer to the object's "this" and not the private methods's "this"
function Foo() {
var self = this;
self.bar = null;
function baz() {
// using this here would refer to baz's scope, so self to the rescue
self.bar = "bazified";
}
}
What I am asking is if compiled swift code would get a memory or processor hit using self to refer object's properties within methods.
Thanks anyway! :-)
fmquaglia
11,060 Pointsfmquaglia
11,060 PointsThanks @stephenwhitfield ! Your answer is clear.