Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial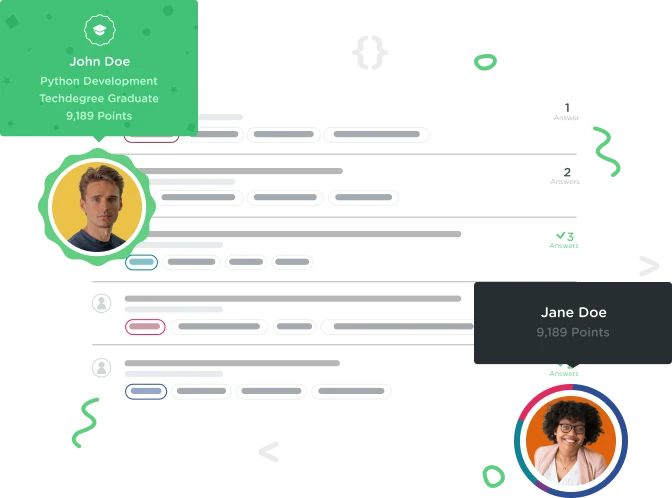
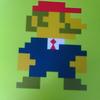
KAZUYA NAKAJIMA
8,851 PointsIs there vulnerability in my code?
I wrote below code as solution for "number guessing game". It seems operating well as I thought. but do you have any suggestion to improve my code? If you see any potential vulnerability which may cause error in my code especially, please point out.
import random
allowed_guess = 5
random_num = random.randint(1, 10)
print('Please guess random number between 1 and 10.')
print('You have 5 times to guess.')
count = 0
while True:
guessed_num = input('> ')
count = count + 1
try:
int_guess = int(guessed_num)
except:
if count >= allowed_guess:
print('You lose! random number was {}.'.format(random_num))
break
print('Your input is not whole number.')
print('You tried {} times. next try!'.format(count))
continue
if not int_guess > 0 or not int_guess < 11:
if count >= allowed_guess:
print('You lose! random number was {}.'.format(random_num))
break
print('Your input is out of range.')
print('You tried {} times. next try!'.format(count))
continue
if int_guess == random_num:
print('You won! random number is {}. You tried {} times.'.format(random_num,count))
break
elif int_guess > random_num:
print('Your guessed number is larger than random number.')
if count >= allowed_guess:
print('You lose! random number was {}.'.format(random_num))
break
else:
print('You tried {} times. next try!'.format(count))
continue
else:
print('Your guessed number is smaller than random number.')
if count >= allowed_guess:
print('You lose! random number was {}.'.format(random_num))
break
else:
print('You tried {} times. next try!'.format(count))
continue
2 Answers

Maxim Andreev
24,529 PointsYou need to use an if statement instead of Try/Except. Try/Except is used for error handling.
Use isinstance( your_variable, int ) to check if your variable is an integer.
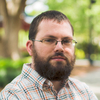
Kenneth Love
Treehouse Guest TeacherI'm not sure you quite get how except
works. That code in there will only be executed if the number they pass in is not convertible to an integer.
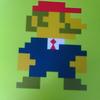
KAZUYA NAKAJIMA
8,851 PointsKenneth: My intention is for this "except" that even player inputs string, count this input as 1 input and goes loop again, not stop the game. so when "count" is lager or equal to 5, this loop stops and when count is smaller to 5, the loop goes to next loop.
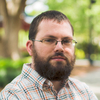
Kenneth Love
Treehouse Guest TeacherAh, I see. I read that too fast. That's a good way of handling it. Good job!
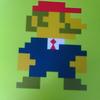
KAZUYA NAKAJIMA
8,851 PointsKenneth: thanks. your way of teaching is very fun. keep it on.
KAZUYA NAKAJIMA
8,851 PointsKAZUYA NAKAJIMA
8,851 PointsMaxim: isinstance(object, classinfo) is new for me thanks for your suggestion and let me investigate this function.