Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial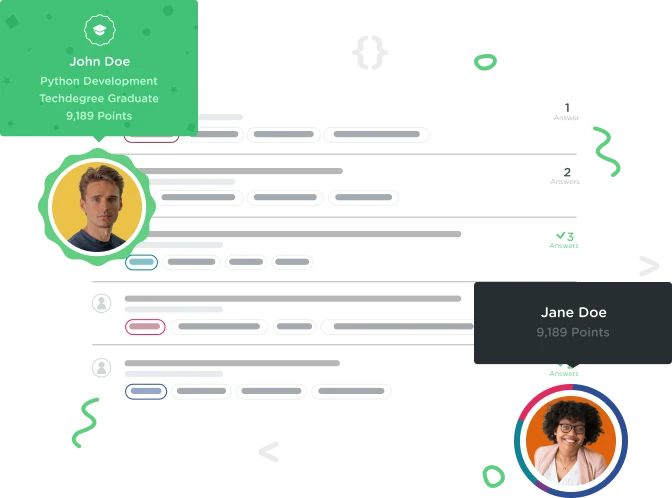

Kishan P
9,921 PointsIs this a DRY code?
var playList = ['I Did It My Way','Respect','Imagine','Born to Run','Louie Louie','Maybellene'];
function printlist( list) {
var listHTML = "<ol>";
for (var i = 0; i < playList.length; i++) {
listHTML = listHTML + "<li>" + list[i] + "</li>" ;
}
listHTML = listHTML + "</ol>";
document.write(listHTML);
}
printlist(playList);
2 Answers
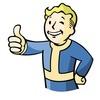
Eric Queen
13,214 Pointsvar playList = [
'I Did It My Way',
'Respect',
'Imagine',
'Born to Run',
'Louie Louie',
'Maybellene'
];
function printlist( list ) {
var listHTML = "<ol>";
for ( var i = 0; i < playList.length; i++ ) {
listHTML = listHTML + "<li>" + list[i] + "</li>" ;
}
listHTML = listHTML + "</ol>";
document.write(listHTML);
}
printlist(playList);
You are definitely not repeating yourself, hence the use of the loop. You did make a mistake by using playList.length in your loop instead of list.length. The way you have it you are hardcoding the length of the list to be printed to just playlist. If you passed in another list that had a different length it would break.
If you were to use this function to create more lists it wouldn't be possible because you are simply writing a list to the DOM. You would want to make it so that you can pass in an argument for the id of the <ol> that you want to add list items to. Otherwise you would be creating another function to do the same thing again, but with a different list. Of course the exact structure and use case for how and what you are creating a list for would really depend on how the function is written. Here is one example that would accessing an <ol> that is already part of the DOM.
var playList = [
'I Did It My Way',
'Respect',
'Imagine',
'Born to Run',
'Louie Louie',
'Maybellene'
];
var myNewList = document.getElementById("newList");
function printlist( list, listId ) {
var listHTML = "";
for ( var i = 0; i < list.length; i++ ) {
listHTML += "<li>" + list[i] + "</li>" ;
}
listId.innerHTML = listHTML;
}
printlist(playList, myNewList);
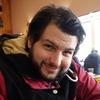
Eric M
11,546 PointsHi Jake,
DRY means Don't Repeat Yourself. This function on its own doesn't repeat anything unnecessarily in terms of lines of code, maintaining this over an entire application is a bit tougher.
If you want to get a little DRYier you can omit a couple of words by using the syntactic sugar of +=
. It's very minor though, and DRY doesn't mean "remove as many characters as you can" so much as "don't create more places to change things than necessary".
Here's the +=
version (excuse my difference in formatting):
function printlist(list)
{
var listHTML = "<ol>";
for (var i = 0; i < list.length; i++)
{
listHTML += "<li>" + list[i] + "</li>";
}
listHTML += "</ol>";
document.write(listHTML);
}

Kishan P
9,921 PointsI don't understand the parameter list in function
i < list.length
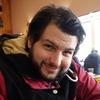
Eric M
11,546 PointsHi Jake,
Eric Queen actually addresses this in his answer. In short, I've just provided the function here, not the code around it, so this function doesn't have any knowledge of the playList
variable.
The function printlist
has one parameter list
. You can see this in the function signature function printlist(list)
The parameter is in the brackets.
So when we call printlist, we pass it one argument, in this case we pass playList
.
Within the printlist function we refer to the parameter list
, because we don't know what's been passed when calling the function.
We might want to run:
printlist(myFirstList);
printlist(mySecondList);
Cheers,
Eric
Eric M
11,546 PointsEric M
11,546 PointsGreat points another really important concent - making things generic and extendable!
Kishan P
9,921 PointsKishan P
9,921 PointsEric Queen I don't understand the parameter list in function
i < list.length