Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial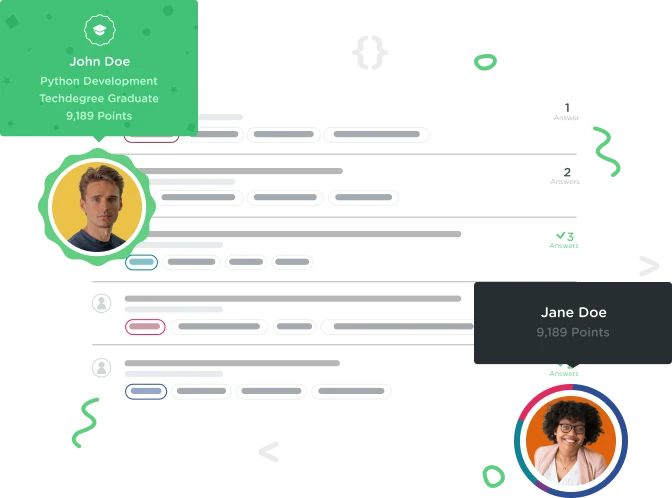
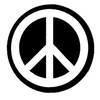
john larson
16,594 Pointsis this a function that accepts two arguments
function max(low, high) { }
2 Answers

Megan Babbitt
7,826 PointsYes the basic formula is :
function name(parameter1, parameter2, parameter3) {
code to be executed
}
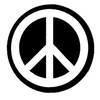
john larson
16,594 Pointslavaughn, I see you used "do and while?"... I gather that is beyond my; if, else and else if. But thank you again for the example.

LaVaughn Haynes
12,397 PointsYeah, I wanted to avoid the if/then/else since I think that you said that you wanted to work that whole thing out for yourself, but it's similar to a while loop that is reversed.
The difference is that the while loop does the test first and then the loop code. The do/while loop does the loop code first, and then the test.
The result is do/while is guaranteed to execute the loop code at least once while it's possible for the while loop to not run the code at all. You might run across these styles of loops when you get to loops but chances are you'll end up using a for loop more than anything which I wont spoil for you.
//both of these count to 10
//one just tests first and then logs, the other logs first and then tests
var x = 1;
while(x <= 10){
console.log(x);
x = x+1;
}
var y = 1;
do{
console.log(y);
y = y+1;
}while(y<= 10);

L MOHAN ARUN
769 PointsHeres one way it does indeed accept two arguments: function max(low, high) { var input = prompt("Enter any number you thought of:"); if (input >= low && input <=high) alert("whatever your input was, it was high enough"); if (input <low || input >high) alert("you are not in range!"); }
Heres second way it does indeed accept two arguments: function max(low,high) { var input=prompt("Enter any number you can think of:"); if (input>low && input<low) { alert("whatever your input was, it was high enough"); } else if (input==high) { alert("hit!"); } else alert("you are not in range!"); }
Heres third way it does indeed accept three arguments: function max(low, high) { alert("Don't think of any number:"); alert("whatever your input was, it was high enough"); }
max(2,9); //output: whatever your input was, it was high enough max(3,9); //output: whatever your input was, it was high enough max(0,1); //only the third max function definition will output //output: whatever your input was, it was high enough max(0,9); //output: whatever your input was, it was high enough var whatishigh=9; is the same as var whatishigh(max(-9,9));
LaVaughn Haynes
12,397 PointsLaVaughn Haynes
12,397 PointsShe's correct. Here's an example of how you could define and use it: