Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial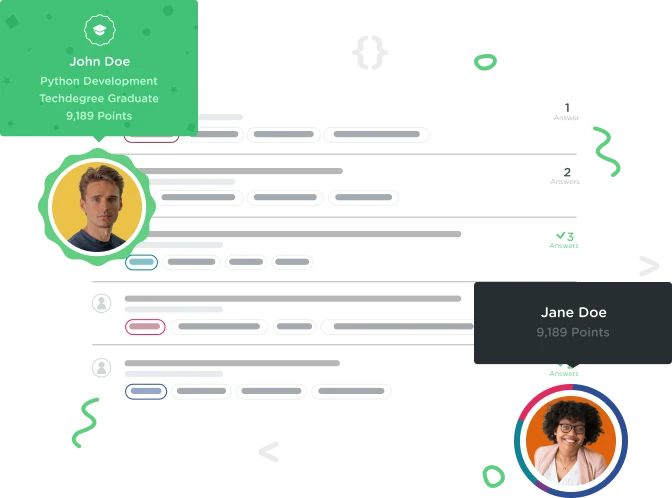
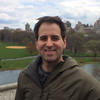
Jordan Mor
Full Stack JavaScript Techdegree Graduate 31,711 PointsIs this a more effective solution to the second challenge in this lesson?
The second challenge in the lesson calls for a final array of all the book titles contained in the array "users".
const users = [
{
name: 'Samir',
age: 27,
favoriteBooks:[
{title: 'The Iliad'},
{title: 'The Brothers Karamazov'}
]
},
{
name: 'Angela',
age: 33,
favoriteBooks:[
{title: 'Tenth of December'},
{title: 'Cloud Atlas'},
{title: 'One Hundred Years of Solitude'}
]
},
{
name: 'Beatrice',
age: 42,
favoriteBooks:[
{title: 'Candide'}
]
}
];
The result should be:
['The Iliad', 'The Brothers Karamazov', 'Tenth of December', 'Cloud Atlas', 'One Hundred Years of Solitude', 'Candide'];
The solution covered in the lesson uses the map method twice (the second map method is used inside the first map method) and then reduces the book arrays into one array with all the book titles.
const books = users
.map(user => user.favoriteBooks.map(book => book.title))
.reduce((arr, titles) => [...arr, ...titles], []);
console.log(books);
Wouldn't it be more effective if we first use the reduce method on the array, and then chain the map method afterwards?
By reducing first, the map method only needs to be used once.
const books = users
.reduce((arr, users) => [...arr, ...user.favoriteBooks], [])
.map(book => book.title);
console.log(books);
2 Answers
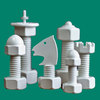
Steven Parker
231,268 PointsSometimes the examples aren't the absolutely most concise or efficient solutions, but then the objective is to illustrate the principles and methods of the lesson.
Plus, that allows for the fun of optimizing it further. And a quick benchmark test shows that you improved the efficiency of the solution by about 26%. Good job!
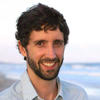
Brenden Seidel
16,890 PointsWas wondering the same thing! I did similar to your solution, but just put the map in the reduce callback:
const books = users.reduce((arr, user) => [...arr, ...user.favoriteBooks.map(book => book.title)], []);
Jordan Mor
Full Stack JavaScript Techdegree Graduate 31,711 PointsJordan Mor
Full Stack JavaScript Techdegree Graduate 31,711 PointsThanks! Still learning, so I wasn't sure if there was some reason the solution covered in the lesson was preferable and I just didn't fully understand the methods yet. And you're right! It was fun optimizing the solution. Just wanted to be sure that's what I did and I wasn't missing something.